Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
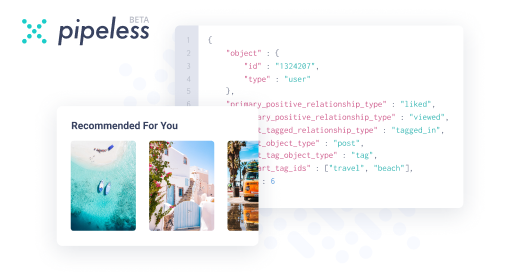
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "event driven"
-
Still trying to get good.
The requirements are forever shifting, and so do the applied paradigms.
I think the first layer is learning about each paradigm.
You learn 5-10 languages/technologies, get a feeling for procedural/functional/OOP programming. You mess around with some electronics engineering, write a bit of assembly. You write an ugly GTK program, an Android todo app, check how OpenGL works. You learn about relational models, about graph databases, time series storage and key value caches. You learn about networking and protocols. You void the warranty of all the devices in your house at some point. You develop preferences for languages and systems. For certain periods of time, you even become an insufferable fanboy who claims that all databases should be replaced by MongoDB, or all applications should be written in C# -- no exceptions in your mind are possible, because you found the Perfect Thing. Temporarily.
Eventually, you get to the second layer: Instead of being a champion for a single cause, you start to see patterns of applicability.
You might have grown to prefer serverless microservice architectures driven by pub/sub event busses, but realize that some MVC framework is probably more suitable for a 5-employee company. You realize that development is not just about picking the best language and best architecture -- It's about pros and cons for every situation. You start to value consistency over hard rules. You realize that even respected books about computer science can sometimes contain lies -- or represent solutions which are only applicable to "spherical cows in a vacuum".
Then you get to the third layer: Which is about orchestrating migrations between paradigms without creating a bigger mess.
Your company started with a tiny MVC webshop written in PHP. There are now 300 employees and a few million lines of code, the framework more often gets in the way than it helps, the database is terribly strained. Big rewrite? Gradual refactor? Introduce new languages within the company or stick with what people know? Educate people about paradigms which might be more suitable, but which will feel unfamiliar? What leads to a better product, someone who is experienced with PHP, or someone just learning to use Typescript?
All that theoretical knowledge about superior paradigms won't help you now -- No clean slates! You have to build a skyscraper city to replace a swamp village while keeping the economy running, together with builders who have no clue what concrete even looks like. You might think "I'll throw my superior engineering against this, no harm done if it doesn't stick", but 9 out of 10 times that will just end in a mix of concrete rubble, corpses and mud.
I think I'm somewhere between 2 and 3.
I think I have most of the important knowledge about a wide array of languages, technologies and architectures.
I think I know how to come to a conclusion about what to use in which scenario -- most of the time.
But dealing with a giant legacy mess, transforming things into something better, without creating an ugly amalgamation of old and new systems blended together into an even bigger abomination? Nah, I don't think I'm fully there yet.8 -
I've optimised so many things in my time I can't remember most of them.
Most recently, something had to be the equivalent off `"literal" LIKE column` with a million rows to compare. It would take around a second average each literal to lookup for a service that needs to be high load and low latency. This isn't an easy case to optimise, many people would consider it impossible.
It took my a couple of hours to reverse engineer the data and implement a few hundred line implementation that would look it up in 1ms average with the worst possible case being very rare and not too distant from this.
In another case there was a lookup of arbitrary time spans that most people would not bother to cache because the input parameters are too short lived and variable to make a difference. I replaced the 50000+ line application acting as a middle man between the application and database with 500 lines of code that did the look up faster and was able to implement a reasonable caching strategy. This dropped resource consumption by a minimum of factor of ten at least. Misses were cheaper and it was able to cache most cases. It also involved modifying the client library in C to stop it unnecessarily wrapping primitives in objects to the high level language which was causing it to consume excessive amounts of memory when processing huge data streams.
Another system would download a huge data set for every point of sale constantly, then parse and apply it. It had to reflect changes quickly but would download the whole dataset each time containing hundreds of thousands of rows. I whipped up a system so that a single server (barring redundancy) would download it in a loop, parse it using C which was much faster than the traditional interpreted language, then use a custom data differential format, TCP data streaming protocol, binary serialisation and LZMA compression to pipe it down to points of sale. This protocol also used versioning for catchup and differential combination for additional reduction in size. It went from being 30 seconds to a few minutes behind to using able to keep up to with in a second of changes. It was also using so much bandwidth that it would reach the limit on ADSL connections then get throttled. I looked at the traffic stats after and it dropped from dozens of terabytes a month to around a gigabyte or so a month for several hundred machines. The drop in the graphs you'd think all the machines had been turned off as that's what it looked like. It could now happily run over GPRS or 56K.
I was working on a project with a lot of data and noticed these huge tables and horrible queries. The tables were all the results of queries. Someone wrote terrible SQL then to optimise it ran it in the background with all possible variable values then store the results of joins and aggregates into new tables. On top of those tables they wrote more SQL. I wrote some new queries and query generation that wiped out thousands of lines of code immediately and operated on the original tables taking things down from 30GB and rapidly climbing to a couple GB.
Another time a piece of mathematics had to generate all possible permutations and the existing solution was factorial. I worked out how to optimise it to run n*n which believe it or not made the world of difference. Went from hardly handling anything to handling anything thrown at it. It was nice trying to get people to "freeze the system now".
I build my own frontend systems (admittedly rushed) that do what angular/react/vue aim for but with higher (maximum) performance including an in memory data base to back the UI that had layered event driven indexes and could handle referential integrity (overlay on the database only revealing items with valid integrity) or reordering and reposition events very rapidly using a custom AVL tree. You could layer indexes over it (data inheritance) that could be partial and dynamic.
So many times have I optimised things on automatic just cleaning up code normally. Hundreds, thousands of optimisations. It's what makes my clock tick.4 -
TL;DR you suck, I suck and everybody sucks, deal with it....
------------------------------------
Let me let off some steam, since I've had enough of people hating on languages "just because"
Every language has it's drawbacks and quirks, BUT they have their strengths also. Saying "I hate {language}" is just you being and ignorant prick and probably your head is so far up your ass that you look like an ass hat. With that being said, every language is either good or bad depending on the developer writing in it. Let's give you an example:
If I ware to give you a brick and ask you to put a nail in a plank, can you do it? Yes, it will be easier if you do it with a hammer, but you have a brick, so hammer is out of the question. If you hit your thumb while doing it... well... sorry, but it is not the bricks fault - it is YOU!
JavaScript, yes it has a whole lot of problems, but it works, you can do a ton of stuff and does a good job at that, it is evolving through node and typescript (and others, just a personal pref), BUT if you used js when you ware debugging that jquery (1.0) plugin written in the free time of a 13 yo, who copy pasted a bunch from SO, well, it is not js' problem - deal with it. Same goes for PHP, i've been there where you had a single `index.php` with bazillion lines of code, did a bunch of eval and it was called MVC, but it also is evolving.. thing is all languages allow you to do some dumb stuff so YOU have to be responsible to not fuck it up (which you always DO btw, we all do). Difference is PHP/JS roll with it because the assumption is that you know what you are doing, which again - newsflash - you don't.
More or less I would blame that shit on businesses which decided to go with undergrads to save money instead of investing in their product, hell, I am in a major company that does not invest that doesn't care a whole lot about dev /tech stuff and now everybody's mother is an engineer - they care about money, because investors care about money (ROI) and because clean code does not pay the bills, but money does.
If we get all of the good practices and apply them to each language every one of them has it's place, that is why there is no "The Language", even if there was, we STILL ware going to fuck it up and probably it was going to be even worse than where we are now.
Study, improve, rinse and repeat... There are SENIORS and LEADS out there that are about 25-30 and have no fucking clue about the language, because they have stuck up their heads up the ass of frameworks and refuse to take a breath of clean air and consider something different than their dogmatic framework "way" of doing things.. That is the result you are seeing. Let me give you a fresh example to illustrate where I am at atm:
Le me works with ZendFramework 2.3-2.5 (why not, which is PHP5+ running on PHP7 [fancy, eh]), and little me writes a module for said project, and tries to contain it in its own space, i.e not touching anything outside of the folder of the module so it is SELF-CONTAINED (see, practices), during 2-3-4 iterations of code review, I've had to modify 4 different modules with `if (somthing === self::SOMETHING_TYPE)` as requested by my TL, which resulted in me not covering 3 use-cases after the changes and not adding a new event (the fw is event-driven, cuz.. reasons) so I have to use a bunch of ifs in the code, to check a config value and do shit. That is the way of I am asked to do things I hate what I've done and the fact that because of CR I have lost case-coverage, a week of work and the same TL will be on my ass on monday that things are now "perfect".
The biggest things is "we care about convention and code style"... right.... That is not because of the language, not because of me, not because of the framework - it is some dude's opinion that you hate, not the language.
New stuff are better, reinventing the wheel is also good, if it wasn't you would've had a few stone circular things on your car and things ware going to be like that - we need to try and try, that is the only way we actually learn shit.
Until things change in the trade, we will be on the same boat, complaining about the same shit over and over, you and me won't be alive probably but things will not change a bit.
We live in a place where state is considered good, god objects necessary (can you believe it, I've got kudos for using the term 'God Object'... yep, let that sink in). If you really hate something, please, oh god I beg you, show me how you will do it better and I will shake your hand and buy you a beer, but until then, please keep your ass-hurt fanboy opinion to your self, no one gives a shit about what you think, we will die and the world will not notice...6 -
TL;DR I want to watch videos faster.
Siraj Raval, a YouTuber who produces videos about artificial intelligence and the concepts behind it (not the buzz-shit-type 😜), once said in one of his videos that he learned to watch videos three times faster.
This man has truly changed my life in many ways.😯
I've started with watching videos with slight multipliers. Around half a year later I learned to watch videos with a multiplier between 1.75 and 2.5.
It's really incredible how much time one can save like this.
25min of free time? Let me just watch that 40min talk about event-driven programming real quick!
Don't do that with gameplays, series, anime and other types of videos 😏 you watch for entertainment because it kinda defies the concept of entertainment.
Link to the video: https://youtu.be/nxWfZP6eslM
Papa tutu tutu tu Wawa:
https://youtu.be/6PJIF_KNvqQ14 -
<rant>
Don't fucking tell me to move business logic from the service to the controller. Don't fucking tell me it will enable an "event driven architecture." Don't fucking use Angular for this project if you're just gonna shit on best practices and write convoluted, messy, inconsistent code and force your coworkers to do the same!
</rant> -
I have a VP constantly harassing my people about some reports that we need to do as per federal law.
The thing is, these live inside of such system that I get to see exactly how many "hits" they get on a yearly basis. The only traffic we have on those sections is of people going ahead and putting the information from our reports there.
That's it, literally. Our user base does not go there. Federal agencies do not go there. No one gives two blips of shit about those sections. Yet she continuously acts like they are the most important thing in the fucking world. To make it better, I was told not to generate actual analytical data from said reports, since people with PHDs will come down on me to ask me who the fuck do I think I am from gauging them with such systems. So shit is a mute point on all fucking accounts.
I told my VP I can generate traffic information to let them know that shit is not really the most important thing in the fucking universe. His eyes glowed.
I don't want to see head rolls, but from staying till the next morning awake trying to give the best to our userbase, and just to be called out on shit like this as if I did not do enough for our people just.....well....it fucking hits man.
The worse part was me literally getting 30 minutes of sitting down after an all nighter, doing something for my users, to get to a meeting the next morning (I should not have driven there honestly) to hear this bitch complain about us not doing enough or not caring or whatever other bullshit she would spew.
I was livid, lack of sleep makes me dangerous. I turned to say something when my boss stopped me and took care of business. I seriously love this man. By all accounts and generational gaps a boomer, but one of the few good golden ones.
I just hate how unappreciated the realm of software development is by people that think that our shit is as simple as making a fucking powerpoint presentation.
Consolidate that with a director from another department taking all fucking glory during a major event of an application that I built by myself with 2 fucking weeks of no sleeping. And shit just gets glorious.
I have considered moving to other places, and heck, have gotten amazing offers, what with having a degree with a big fucking GPA and having the credentials of a senior, lead, full stack and manager role, the sky is the limit. But i know that if I leave then my users suffer, and I just can't fucking have that.
I have heard them speaking about doing something with X app that I built (with my department) I have even heard one of them saying "how is this made?" and a part of me hoped that it would be a good time to grab them and tell them of the field and the things that they can do. But I don't like announcing myself that way, always seemed to presumptuous, so I just smile, fuck yeah, my users are doing their thing with what I built to better their lives, what more can I have?
I have gotten criticisms from them, one recognized me, told me about his pain points and how it makes it hard for him to do what he must. Getting the data from the user base in an effort to make shit better for them drives me, my challenge being "how about this? better eh?"
But fucking execs man, think only of themselves, not the users, they forget about the users. Much like a shitty rock band forgetting about the music, about the fans.
I can't let that slide. But this fucking field. I sometimes fucking hate it, and I hate it because of the normies that don't understand and do not want to understand.
I do way too much, my guys do way too much and all I want is for the recognition to go to them. They do not need the ego boost, but to see my guys sitting in a meeting in which some dumb fuck is trying to drill us for taking to long, not doing something and what not, it fucking pisses me off. As their boss I always stand up and tell bitches off, but instead of learning, the bitches just keep pressing on their already defeated points.
Everything in human life gets fucking erradicated by: humans. People really do fucking suck.
I sometimes wish to go back, redo my diesel tech license and just work there, where I think one would be better of talking to an engine. But no, even then you get people, you have to interact with people, deal with people, and I am so far up my game and in my field that starting from scratch is a fucking mute point.
Maybe I need to keep fucking with stocks, get rich and just keep investing on bullshit. Whatever the fuck it takes me from having to feel the urge to choke a motherfucker in public.1 -
Over the past 2 months I have interviewed with several companies and 2 of them stood out at rejecting me. Let's call them Company A, and Company B!
> I know right? Developers are bad at naming!
I guess part of it is my fault too! I am old and slow. Doesn't like competitive programming and already forgot most of how to answer algorithm question. I can't even answer some of the algorithm question I've flawlessly answered back when I was fresh out of University.
## Company A
When I got chance to interview at Company A, they require me to answer HackerRank style interview. It's my first time in nearly a decade of working in the industry to feel like I'm in a classroom exam again. I hate it, and I deliberately voiced my distaste to the answers comment:
// Paraphrasing
// I'm sorry, I'm dumb!
// I never faced anything like this in real world work...
// ......
But guess what? My answer still pass the score, have a call with their VP, which proceed to have another call with their Lead Engineer.
Talked about my experience with Event Driven System and CQRS+ES and they decided that I am:
- Arrogant
- Too RND in my tech stack
- And overkill in CQRS+ES
And decided they don't need me.
They hate me for having a headstrong personality which translates as Arrogance to the perceiving end.
## Company B
Another HackerRank style interview. Guess I passed their score this time without me typing some strong comment and proceed to have another test with their Lead Engineer.
This time they want 5 question answered in google docs within 60 minutes.
Two of them stood out to me for being impossible to work on 12 minutes (60 / 5 if you're wondering). Or maybe I'm just old and dumb?!
The others are just questions copied word for word from Geeks For Geeks.
One of the question requires me to write a password brute force attack to an imaginary API.
The other requires me to find a combination of math `+` or `-` operation from `a strings of numbers` that results in `a number`.
My `Arrogance` kicks in and I start typing a comment
// Paraphrasing
// I am sorry but I feel this is impossible for me to think of in 12 minutes
// (60 / 5 if you're wondering)
// But I know you guys got this question from Rosseta Code!
// Here's the link, but I don't know the logic behind it
See? I've worked on this question back when I was still a University student and remember where to look at.
Unsurprisingly, I've heard the feedback that I was rejected although I've answered one of their question `FLAWLESSLY`. I know they are being sarcastic at this point. haha.
---
I was trying to be honest about what I can and can't do in the `N` minutes timeframe and the Industry hates me.
I guess The Industry love people who can grind `GFG` or other algorithm websites, remember the solutions out of their head, and quietly answer their `genuinely original question` without pointing the flaws back at them.9 -
(long post is long)
This one is for the .net folks. After evaluating the technology top to bottom and even reimplementing several examples I commonly use for smoke testing new technology, I'm just going to call it:
Blazor is the next Silverlight.
It's just beyond the pale in terms of being architecturally flawed, and yet they're rushing it out as hard as possible to coincide with the .Net 5 rebranding silo extravaganza. We are officially entering round 3 of "sacrifice .Net on the altar of enterprise comfort." Get excited.
Since we've arrived here, I can only assume the Asp.net Ajax fiasco is far enough in the past that a new generation of devs doesn't recall its inherent catastrophic weaknesses. The architecture was this:
1. Create a component as a "WebUserControl"
2. Any time a bound DOM operation occurs from user interaction, send a payload back to the server
3. The server runs the code to process the event; it spits back more HTML
Some client-side js then dutifully updates the UI by unceremoniously stuffing the markup into an element's innerHTML property like so much sausage.
If you understand that, you've adequately understood how Blazor works. There's some optimization like signalR WebSockets for update streaming (the first and only time most blazor devs will ever use WebSockets, I even see developers claiming that they're "using SignalR, Idserver4, gRPC, etc." because the template seeds it for them. The hubris.), but that's the gist. The astute viewer will have noticed a few things here, including the disconnect between repaints, inability to blend update operations and transitions, and the potential for absolutely obliterative, connection-volatile, abusive transactional logic flying back and forth to the server. It's the bring out your dead approach to seeing how much of your IT budget is dedicated to paying for bandwidth and CPU time.
Blazor goes a step further in the server-side render scenario and sends every DOM event it binds to the server for processing. These include millisecond-scale events like scroll, which, at least according to GitHub issues, devs are quickly realizing requires debouncing, though they aren't quite sure how to accomplish that. Since this immediately becomes an issue with tickets saying things like, "scroll event crater server, Ugg need help! You said Blazorclub good. Ugg believe, Ugg wants reparations!" the team chooses a great answer to many problems for the wrong reasons:
gRPC
For those who aren't familiar, gRPC has a substantial amount of compression primarily courtesy of a rather excellent binary format developed by Google. Who needs the Quickie Mart, or indeed a sound markup delivery and view strategy when you can compress the shit out of the payload and ignore the problem. (Shhh, I hear you back there, no spoilers. What will happen when even that compression ceases to cut it, indeed). One might look at all this inductive-reasoning-as-development and ask themselves, "butwai?!" The reason is that the server-side story is just a way to buy time to flesh out the even more fundamentally broken browser-side story. To explain that, we need a little perspective.
The relationship between Microsoft and it's enterprise customers is your typical mutually abusive co-dependent relationship. Microsoft goes through phases of tacit disinterest, where it virtually ignores them. And rightly so, the enterprise customers tend to be weaksauce, mono-platform, mono-language types who come to work, collect a paycheck, and go home. They want to suckle on the teat of the vendor that enables them to get a plug and play experience for delivering their internal systems.
And that's fine. But it's also dull; it's the spouse that lets themselves go, it's the girlfriend in the distracted boyfriend meme. Those aren't the people who keep your platform relevant and competitive. For Microsoft, that crowd has always been the exploratory end of the developer community: alt.net, and more recently, the dotnet core community (StackOverflow 2020's most loved platform, for the haters). Alt.net seeded every competitive advantage the dotnet ecosystem has, and dotnet core capitalized on. Like DI? You're welcome. Are you enjoying MVC? Your gratitude is understood. Cool serializers, gRPC/protobuff, 1st class APIs, metadata-driven clients, code generation, micro ORMs, etc., etc., et al. Dear enterpriseur, you are fucking welcome.
Anyways, b2blazor. So, the front end (Blazor WebAssembly) story begins with the average enterprise FOMO. When enterprises get FOMO, they start to Karen/Kevin super hard, slinging around money, privilege, premiere support tickets, etc. until Microsoft, the distracted boyfriend, eventually turns back and says, "sorry babe, wut was that?" You know, shit like managers unironically looking at cloud reps and demanding to know if "you can handle our load!" Meanwhile, any actual engineer hides under the table facepalming and trying not to die from embarrassment.36 -
4 years ago I made a personal goal/plan to be a full stack developer. Meaning a good understanding of any development between os level code and web/front end user experience.
Over the years this term 'full stack' has been abused greatly and now basically means 'a javascript developer that generally knows what they are talking about'.
So now, devRant collective I ask you. What do you call a developer with good skills in:
- os level code (c, c++ and os apis)
- database level tech (advanced querying and db aglo/modeling)
- software architecture
- application level (workflow and business logic)
- transport level (protocol design and usage)
- front end tech (graphics programming and event driven paradigm)
- user experience14 -
Rails. Fucking rails...
God damn monoliths, built by a cowboy coder.
Every one I have ever worked with becomes (or already is) a house of fucking cards that will blow over at the slightest gust of wind.
The worst part is that you always hear the same justifications from rails developers, then after they convince the higher-ups that “we will build it right, not like those other monoliths” we find ourselves F’ed right in the A a few months later.
It’s this frustration that lead me to MUCH better paradigms like Microservices, Event-Sourcing, CQRS, Domain Driven Design and the like.
When someone says “our backend is in rails” my first response is “so when are you replacing it?”8 -
So happy about being about to convince management that we needed a large refactor, due to requirements change, and since the code architecture from the beginning had boundaries built before knowing all the requirements...
pulled the shame on us, this is a learning lesson card.. blah blah blah
Also explained we need to implement an RTOS, and make the system event driven... which then a stupid programmer said you mean interrupt driven ... and management lost their minds... ( bad memories of poorly executed interrupts in the past).... had to bring everyone back down to earth.. explained yes it’s interrupt driven, but interrupt driven properly unlike in the past (prior to me)... the fuck didn’t properly prioritize the interrupts and did WAYYY too much in the interrupts.
Explained we will be implementing interrupts along side DMA, and literally no message could be lost in normal execution.. and explained polling the old way along side no RTOS, Wastes power, CPU resources and throws timing off.
Same fucker spoke up and said how the fuck You supposed to do timing, all the timing will be further off... I said wrong, in this system .. unlike yours, this is discreet timing potential and accurate as fuck... unlike your round robin while loop of death.
Anyway they gave me 3 weeks.. and the system out performs, and is more power efficient than the older model.
The interrupting developer, now gives me way more respect...4 -
So I’m like 90% certain 10% of my app should be event sourced, and the other 90% CRUD based (but event driven).
However after trying to tackle this from a variety of angles I’m 100% ready to jump off a building
So I’m taking a break and eating a fucking donut1 -
In my school we had a CS lab and we were supposed to do lab assignments.
I had a book which gave a basic introduction on event driven programming, and introduced me to two new functions which I couldn't have ever known(I got internet in 2013) if I had gone with just the curriculum, kbhit() and gotoxy(). With this new knowledge I created my first 2d game. And that feeling of creating something no one expects and something fun, which also gets you attention of the whole room(Nothing like that was ever created in that lab, it was a shitty school), made me realize that this is what I want to do for the rest of my life. 😃 -
Question.. architecting a large system. I’ve broken it down to microservices for the DB and rest API / gateway
I want there to be some some processes that run continuously not event driven via rest. Say analytics for example what is the best way todo that? Just another service running on on a server? And said service has its own API? That when the other rest APIs are called could then hop and call the new service?
Or say we had a PDF upload via rest should that service then do the parsing before uploading to DB .. or should the rest api that does the uploading then call another rest api to another service dedicated todo the parsing and uploading to the db?
I think the bigger way to explain the question is the encapsulation between DAL.. data access layer which I have existing.. but then there’s the BLL .. buisness logic layer which I don’t know if it should have its own APIs via own microservices running in the background.10 -
A very long rant.. but I'm looking to share some experiences, maybe a different perspective.. huge changes at the company.
So my company is starting our microservices journey (we have a 359 retail websites at this moment)
First question was: What to build first?
The first thing we had to do was to decide what we wanted to build as our first microservice. We went looking for a microservice that can be used read only, consumers could easily implement without overhauling production software and is isolated from other processes.
We’ve ended up with building a catalog service as our first microservice. That catalog service provides consumers of the microservice information of our catalog and its most essential information about items in the catalog.
By starting with building the catalog service the team could focus on building the microservice without any time pressure. The initial functionalities of the catalog service were being created to replace existing functionality which were working fine.
Because we choose such an isolated functionality we were able to introduce the new catalog service into production step by step. Instead of replacing the search functionality of the webshops using a big-bang approach, we choose A/B split testing to measure our changes and gradually increase the load of the microservice.
Next step: Choosing a datastore
The search engine that was in production when we started this project was making user of Solr. Due to the use of Lucene it was performing very well as a search engine, but from engineering perspective it lacked some functionalities. It came short if you wanted to run it in a cluster environment, configuring it was hard and not user friendly and last but not least, development of Solr seemed to be grinded to a halt.
Elasticsearch started entering the scene as a competitor for Solr and brought interesting features. Still using Lucene, which we were happy with, it was build with clustering in mind and being provided out of the box. Managing Elasticsearch was easy since there are REST APIs for configuration and as a fallback there are YAML configurations available.
We decided to use Elasticsearch since it provides us the strengths and capabilities of Lucene with the added joy of easy configuration, clustering and a lively community driving the project.
Even bigger challenge? Which programming language will we use
The team responsible for developing this first microservice consists out of a group web developers. So when looking for a programming language for the microservice, we went searching for a language close to their hearts and expertise. At that time a typical web developer at least had knowledge of PHP and Javascript.
What we’ve noticed during researching various languages is that almost all actions done by the catalog service will boil down to the following paradigm:
- Execute a HTTP call to fetch some JSON
- Transform JSON to a desired output
- Respond with the transformed JSON
Actions that easily can be done in a parallel and asynchronous manner and mainly consists out of transforming JSON from the source to a desired output. The programming language used for the catalog service should hold strong qualifications for those kind of actions.
Another thing to notice is that some functionalities that will be built using the catalog service will result into a high level of concurrent requests. For example the type-ahead functionality will trigger several requests to the catalog service per usage of a user.
To us, PHP and .NET at that time weren’t sufficient enough to us for building the catalog service based on the requirements we’ve set. Eventually we’ve decided to use Node.js which is better suited for the things we are looking for as described earlier. Node.js provides a non-blocking I/O model and being event driven helps us developing a high performance microservice.
The leap to start programming Node.js is relatively small since it basically is Javascript. A language that is familiar for the developers around that time. While Node.js is displaying some new concepts it is relatively easy for a developer to start using it.
The beauty of microservices and the isolation it provides, is that you can choose the best tool for that particular microservice. Not all microservices will be developed using Node.js and Elasticsearch. All kinds of combinations might arise and this is what makes the microservices architecture so flexible.
Even when Node.js or Elasticsearch turns out to be a bad choice for the catalog service it is relatively easy to switch that choice for magic ‘X’ or component ‘Z’. By focussing on creating a solid API the components that are driving that API don’t matter that much. It should do what you ask of it and when it is lacking you just replace it.
Many more headaches to come later this year ;)3 -
Made me think and treat other people like disposable objects.
I also try to send as few packets to them as a result, u kno', to keeping the noise down.
Nah, just kidding.
But it has given me a solid foundation and framework for understanding for understanding so much in life..
Programming have also granted me something I continue enjoying and that I don't grow bored of quickly...
Particularly object oriented and event driven development have given me a pretty good ground to support me, on my personal endeavors onto noeroscience and understanding of the human mind..
Just for fun and curiosity tho :) -
Sydochen has posted a rant where he is nt really sure why people hate Java, and I decided to publicly post my explanation of this phenomenon, please, from my point of view.
So there is this quite large domain, on which one or two academical studies are built, such as business informatics and applied system engineering which I find extremely interesting and fun, that is called, ironically, SAD. And then there are videos on youtube, by programmers who just can't settle the fuck down. Those videos I am talking about are rants about OOP in general, which, as we all know, is a huge part of studies in the aforementioned domain. What these people are even talking about?
Absolutely obvious, there is no sense in making a software in a linear pattern. Since Bikelsoft has conveniently patched consumers up with GUI based software, the core concept of which is EDP (event driven programming or alternatively, at least OS events queue-ing), the completely functional, linear approach in such environment does not make much sense in terms of the maintainability of the software. Uhm, raise your hand if you ever tried to linearly build a complex GUI system in a single function call on GTK, which does allow you to disregard any responsibility separation pattern of SAD, such as long loved MVC...
Additionally, OOP is mandatory in business because it does allow us to mount abstraction levels and encapsulate actual dataflow behind them, which, of course, lowers the costs of the development.
What happy programmers are talking about usually is the complexity of the task of doing the OOP right in the sense of an overflow of straight composition classes (that do nothing but forward data from lower to upper abstraction levels and vice versa) and the situation of responsibility chain break (this is when a class from lower level directly!! notifies a class of a higher level about something ignoring the fact that there is a chain of other classes between them). And that's it. These guys also do vouch for functional programming, and it's a completely different argument, and there is no reason not to do it in algorithmical, implementational part of the project, of course, but yeah...
So where does Java kick in you think?
Well, guess what language popularized programming in general and OOP in particular. Java is doing a lot of things in a modern way. Of course, if it's 1995 outside *lenny face*. Yeah, fuck AOT, fuck memory management responsibility, all to the maximum towards solving the real applicative tasks.
Have you ever tried to learn to apply Text Watchers in Android with Java? Then you know about inline overloading and inline abstract class implementation. This is not right. This reduces readability and reusability.
Have you ever used Volley on Android? Newbies to Android programming surely should have. Quite verbose boilerplate in google docs, huh?
Have you seen intents? The Android API is, little said, messy with all the support libs and Context class ancestors. Remember how many times the language has helped you to properly orient in all of this hierarchy, when overloading method declaration requires you to use 2 lines instead of 1. Too verbose, too hesitant, distracting - that's what the lang and the api is. Fucking toString() is hilarious. Reference comparison is unintuitive. Obviously poor practices are not banned. Ancient tools. Import hell. Slow evolution.
C# has ripped Java off like an utter cunt, yet it's a piece of cake to maintain a solid patternization and structure, and keep your code clean and readable. Yet, Cs6 already was okay featuring optionally nullable fields and safe optional dereferencing, while we get finally get lambda expressions in J8, in 20-fucking-14.
Java did good back then, but when we joke about dumb indian developers, they are coding it in Java. So yeah.
To sum up, it's easy to make code unreadable with Java, and Java is a tool with which developers usually disregard the patterns of SAD. -
I am working on an event driven system that uses a message bus and has a few services that talk to each other asynchronously via the bus.
I'm writing in memory integration tests for one of those services, but I just realised the fundamental flaw here with such tests. I only have 1 application running, but I need several. This is quite a serious flaw I should have seen before.
Anyone else tried integration testing event driven distributed services? I imagine all I can do is stub the message broker...8 -
I'd like to hear opinions from experienced devs/software architects... Referencing my two previous rants, the imposter's has been strong today. And I really don't know how to feel about the possible solution I've come up with... Adding the new feature as a microservice for an otherwise monolithic application 🙄 is that a sane idea?
The thing is I need to have a subscription type event-driven mechanism and since we're listening to service bus messages from another cloud provider, I apparently can't just have a serverless function to do the job, so unless there's a better option, I need a microservice with the subscription that can then invoke a serverless function to actually do what needs to be done. That's my idea, but I'm far from sure this is the best way...1