Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
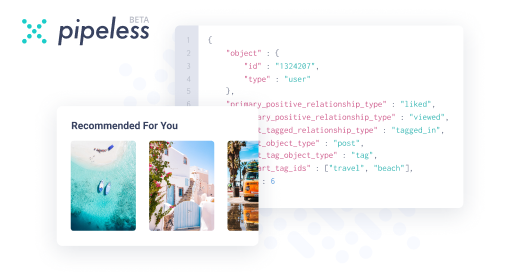
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "line length"
-
Started being a Teaching Assistant for Intro to Programming at the uni I study at a while ago and, although it's not entirely my piece of cake, here are some "highlights":
* students were asked to use functions, so someone was ingenious (laughed my ass off for this one):
def all_lines(input):
all_lines =input
return all_lines
* "you need to use functions" part 2
*moves the whole code from main to a function*
* for Math-related coding assignments, someone was always reading the input as a string and parsing it, instead of reading it as numbers, and was incredibly surprised that he can do the latter "I always thought you can't read numbers! Technology has gone so far!"
* for an assignment requiring a class with 3 private variables, someone actually declared each variable needed as a vector and was handling all these 3 vectors as 3D matrices
* because the lecturer specified that the length of the program does not matter, as long as it does its job and is well-written, someone wrote a 100-lines program on one single line
* someone was spamming me with emails to tell me that the grade I gave them was unfair (on the reason that it was directly crashing when run), because it was running on their machine (they included pictures), but was not running on mine, because "my Python version was expired". They sent at least 20 emails in less than 2h
* "But if it works, why do I still have to make it look better and more understandable?"
* "can't we assume the input is always going to be correct? Who'd want to type in garbage?"
* *writes 10 if-statements that could be basically replaced by one for-loop*
"okay, here, you can use a for-loop"
*writes the for loop, includes all the if-statements from before, one for each of the 10 values the for-loop variable gets*
* this picture
N.B.: depending on how many others I remember, I may include them in the comments afterwards19 -
Ladies and gentlemen, prepare yourselves for a rant with a capital R, this is gonna be a long one.
Our story begins well over a year ago while I was still in university and things such as "professionalism" and "doing your job" are suggestions and not something you do to not get fired. We had multiple courses with large group projects that semester and the amount of reliable people I knew that weren't behind a year and in different courses was getting dangerously low. There were three of us who are friends (the other two henceforth known as Ms Reliable and the Enabler) and these projects were for five people minimum. The Enabler knew a couple of people who we could include, so we trusted her and we let them onto the multiple projects we had.
Oh boy, what a mistake that was. They were friends, a guy and a girl. The girl was a good dev, not someone I'd want to interact with out of work but she was fine, and a literal angel compared to the guy. Holy shit this guy. This guy, henceforth referred to as Mr DDTW, is a motherfucking embarrassment to devs everywhere. Lazy. Arrogant. Standards so low they're six feet under. Just to show you the sheer depth of this man's lack of fucks given, he would later reveal that he picked his thesis topic "because it's easy and I don't want to work too hard". I haven't even gotten into the meat of the rant yet and this dude is already raising my blood pressure.
I'll be focusing on one project in particular, a flying vehicle simulator, as this was the one that I was the most involved in and also the one where shit hit the fan hardest. It was a relatively simple-in-concept development project, but the workload was far too much for one person, meaning that we had to apply some rudimentary project management and coordination skills that we had learned to keep the project on track. I quickly became the de-facto PM as I had the best grasp on the project and was doing a lot of the heavy lifting.
The first incident happened while developing a navigation feature. Another teammate had done the basics, all he had to do was use the already-defined interfaces to check where the best place to land would be, taking into account if we had enough power to do so. Mr DDTW's code:
-Wasn't actually an algorithm, just 90 lines of if statements sandwiched between the other teammate's code.
-The if statements were so long that I had to horizontal scroll to see the end, approx 200 characters long per line.
-Could've probably been 20 normal-length lines MAX if he knew what a fucking for loop was.
-Checked about a third of the tiles that it should have because, once again, it's a series of concatenated if statements instead of an actual goddamn algorithm.
-IT DIDN'T FUCKING WORK!
My response was along the lines of "what the fuck is this?". This dipshit is in his final year and I've seen people write better code in their second semester. The rest of the team, his friend included, agreed that this was bad code and that it should be redone properly. The plan was for Mr DDTW to move his code into a new function and then fix it in another branch. Then we could merge it back when it was done. Well, he kept on saying it was done but:
-It still wasn't an algorithm.
-It was still 90 lines.
-They were still 200 characters wide.
-It still only checked a third of the tiles.
-IT STILL DIDN'T FUCKING WORK!
He also had one more task, an infinite loop detection system. He watched while Ms Reliable did the fucking work.
We hit our first of two deadlines successfully. We still didn't have a decent landing function but everything else was nice and polished, and we got graded incredibly well. The other projects had been going alright although the same issue of him not doing shit applied. Ms Reliable and I, seeing the shitstorm that would come if this dude didn't get his act together, lodged a complaint with the professor as a precautionary measure. Little did I know how much that advanced warning would save my ass later on.
Second sprint begins and I'm voted in as the actual PM this time. We have four main tasks, so we assign one person to each and me as a generalist who would take care of the minor tasks as well as help out whoever needed it. This ended up being a lot of reworking and re-abstracting, a lot of helping and, for reasons that nobody ever could have predicted, one of the main tasks.
These main tasks were new features that would need to be integrated, most of which had at least some mutual dependencies. Part of this project involved running our code, which would connect to the professor's test server and solve a server-side navigation problem. The more of these we solved, the better the grade, so understandably we needed an MVP to see if our shit worked on the basic problems and then fix whatever was causing the more advanced ones to fail. We decided to set an internal deadline for this MVP. Guess who didn't reach it?
Hitting the character limit, expect part 2 SOON7 -
I had to open the desktop app to write this because I could never write a rant this long on the app.
This will be a well-informed rebuttal to the "arrays start at 1 in Lua" complaint. If you have ever said or thought that, I guarantee you will learn a lot from this rant and probably enjoy it quite a bit as well.
Just a tiny bit of background information on me: I have a very intimate understanding of Lua and its c API. I have used this language for years and love it dearly.
[START RANT]
"arrays start at 1 in Lua" is factually incorrect because Lua does not have arrays. From their documentation, section 11.1 ("Arrays"), "We implement arrays in Lua simply by indexing tables with integers."
From chapter 2 of the Lua docs, we know there are only 8 types of data in Lua: nil, boolean, number, string, userdata, function, thread, and table
The only unfamiliar thing here might be userdata. "A userdatum offers a raw memory area with no predefined operations in Lua" (section 26.1). Essentially, it's for the API to interact with Lua scripts. The point is, this isn't a fancy term for array.
The misinformation comes from the table type. Let's first explore, at a low level, what an array is. An array, in programming, is a collection of data items all in a line in memory (The OS may not actually put them in a line, but they act as if they are). In most syntaxes, you access an array element similar to:
array[index]
Let's look at c, so we have some solid reference. "array" would be the name of the array, but what it really does is keep track of the starting location in memory of the array. Memory in computers acts like a number. In a very basic sense, the first sector of your RAM is memory location (referred to as an address) 0. "array" would be, for example, address 543745. This is where your data starts. Arrays can only be made up of one type, this is so that each element in that array is EXACTLY the same size. So, this is how indexing an array works. If you know where your array starts, and you know how large each element is, you can find the 6th element by starting at the start of they array and adding 6 times the size of the data in that array.
Tables are incredibly different. The elements of a table are NOT in a line in memory; they're all over the place depending on when you created them (and a lot of other things). Therefore, an array-style index is useless, because you cannot apply the above formula. In the case of a table, you need to perform a lookup: search through all of the elements in the table to find the right one. In Lua, you can do:
a = {1, 5, 9};
a["hello_world"] = "whatever";
a is a table with the length of 4 (the 4th element is "hello_world" with value "whatever"), but a[4] is nil because even though there are 4 items in the table, it looks for something "named" 4, not the 4th element of the table.
This is the difference between indexing and lookups. But you may say,
"Algo! If I do this:
a = {"first", "second", "third"};
print(a[1]);
...then "first" appears in my console!"
Yes, that's correct, in terms of computer science. Lua, because it is a nice language, makes keys in tables optional by automatically giving them an integer value key. This starts at 1. Why? Lets look at that formula for arrays again:
Given array "arr", size of data type "sz", and index "i", find the desired element ("el"):
el = arr + (sz * i)
This NEEDS to start at 0 and not 1 because otherwise, "sz" would always be added to the start address of the array and the first element would ALWAYS be skipped. But in tables, this is not the case, because tables do not have a defined data type size, and this formula is never used. This is why actual arrays are incredibly performant no matter the size, and the larger a table gets, the slower it is.
That felt good to get off my chest. Yes, Lua could start the auto-key at 0, but that might confuse people into thinking tables are arrays... well, I guess there's no avoiding that either way.13 -
Here's a silly side project I've been working on just for fun:
https://soundofco.de/
It "programmatically" creates sound from code (or plain text) on GitHub. I wouldn't call it music, because it sounds kind of horrible almost all of the time :D It's very simple: The length of a line defines the note to be played. Really just a nerdy bit of fun.
Unfortunately I have absolutely zero knowledge in music theory and I have no idea how to make the resulting sounds more pleasant or even melodic. If you're interested in making this better or helping out with feedback, you can find the project at https://github.com/dneustadt/...
Also feel free to share if you find anything on GitHub that makes for some decent sounds :D (I doubt it)12 -
So Friday afternoon is always deployment time at my company. No sure why, but it always fucks us.
Anyways, last Friday, we had this lovely deployment that was missing a key piece. On Wednesday I had tested it, sent out an email(with screenshots) saying "yo, whoever wrote this, this feature is all fucked up." Management said they would handle it.
The response email. 1(out of 20) defects I sent in were not a defect but my error. No further response, so I assume the rest were being looked into.
In a call with bossman, my manager states that the feature is fixed, so I go to check it quickly before the deployment(on Friday).
THERE IS NO FUCKING CODE CHECK-IN. THE DEV BASTARD JUST SAID THAT MY USECASE WAS WRONG, SO MY ENTIRE EMAIL WAS INVALID.
I am currently working on Saturday, as the other guy refuses to see the problem! It is blatant, and I got 3 other people to reproduce to prove I am not crazy!
On top of that, the code makes me want to vomit! I write bad code. This is like a 3rd grader who doesn't know code copy-pasted from stack overflow! There is literally if(A) then B else if(!A) then B! And a for loop which does some shit, and the line after it closes has a second for loop that iterates over the same unaltered set! Why?! On top of that, the second for loop loops until "i" is equal to length-1, then does something! Why loop???
The smartest part of him ran down his Mama's leg when it saw the DNA dad was contributing!
Don't know who is the culprit, and if you happen to see this, I am pissed. I am working on Saturday because you can't check your code or you lied on your resume to get this job, as you are not qualified! Fuck you!15 -
I have a coworker who comments every line of code he writes and it doesn't matter how simple the code is and it drives me crazy when I have to look at it. A real life example:
// Gets the total length of the server name string
var total = serverName.length;6 -
A jr colleague came back from a react.js code camp.
Those hipsters turned the poor kid into a one liner terrorist and buzzword spammer.
It's time to play bad cop and start enforcing line length limit. -
So... did I mention I sometimes hate banks?
But I'll start at the beginning.
In the beginning, the big bang created the universe and evolution created humans, penguins, polar bea... oh well, fuck it, a couple million years fast forward...
Your trusted, local flightless bird walks into a bank to open an account. This, on its own, was a mistake, but opening an online bank account as a minor (which I was before I turned 18, because that was how things worked) was not that easy at the time.
So, yours truly of course signs a contract, binding me to follow the BSI Grundschutz (A basic security standard in Germany, it's not a law, but part of some contracts. It contains basic security advice like "don't run unknown software, install antivirus/firewall, use strong passwords", so it's just a basic prototype for a security policy).
The copy provided with my contract states a minimum password length of 8 (somewhat reasonable if you don't limit yourself to alphanumeric, include the entire UTF 8 standard and so on).
The bank's online banking password length is limited to 5 characters. So... fuck the contract, huh?
Calling support, they claimed that it is a "technical neccessity" (I never state my job when calling a support line. The more skilled people on the other hand notice it sooner or later, the others - why bother telling them) and that it is "stored encrypted". Why they use a nonstandard way of storing and encrypting it and making it that easy to brute-force it... no idea.
However, after three login attempts, the account is blocked, so a brute force attack turns into a DOS attack.
And since the only way to unblock it is to physically appear in a branch, you just would need to hit a couple thousand accounts in a neighbourhood (not a lot if you use bots and know a thing or two about the syntax of IBAN numbers) and fill up all the branches with lots of potential hostages for your planned heist or terrorist attack. Quite useful.
So, after getting nowhere with the support - After suggesting to change my username to something cryptic and insisting that their homegrown, 2FA would prevent attacks. Unless someone would login (which worked without 2FA because the 2FA only is used when moving money), report the card missing, request a new one to a different address and log in with that. Which, you know, is quite likely to happen and be blamed on the customer.
So... I went to cancel my account there - seeing as I could not fulfill my contract as a customer. I've signed to use a minimum password length of 8. I can only use a password length of 5.
Contract void. Sometimes, I love dealing with idiots.
And these people are in charge of billions of money, stock and assets. I think I'll move to... idk, Antarctica?4 -
I've got a HDD, gotta look for any data left on it. I don't need a 1:1 copy of the drive to determine if any data is even there still, and i'm also strapped for space, so I threw together a little 20-line or so Python script to skip over large amounts of empty space so I only have to sift through what little it finds to see if anything's left at all. I wrote it, ran it... and it took a while. Too long. My SSD was floored and RAM was full for no reason. I double-checked my code...
device.seek(0)
device_size = len(device.read())
If you don't get it, this would've tried to load the ENTIRE DRIVE'S CONTENTS into RAM before giving the length. The drive's 128GB or so. That's not happening.
I'm a fucking idiot.
EDIT:
This is what this was for. This ain't ext4. What in the fuck...?11 -
In a programming exam, we had to write a program in 60 minutes, part of which was sorting some strings by length (strings the same length had to be in the same line)... I had like 3 minutes left, so i wrote this beauty:
boolean b = false;
for(int i = 0; i <= 999999; i++){
for(int j = 0; j <= strings.length; j++){
if(i == strings[j].length()){
System.out.print(s + " ");
b = true;
}
}
if(b){
System.out.println();
b = false;
}
}6 -
Fuck you windows 10. Fuck you private keys. Fuck you tortoise git. Fuck you git bash. Fuck you cygwin. Want 3x hours of my life back. Had an auth problem... Had to reinstall all the above on windows to connect to my private repo. Took me 5 minutes to connect after reinstalling all the tools. Grrrrrrr. And I'll never know why it wouldn't connect apart from fatal protocol error: bad line length character..I tried ever stack overflow answer... I nearly bricked my gitlab CE...and it was windows being a motherslut8
-
Well, throughout my life I've never really thought about programming. Then one day during some downtime on a backpacking trip with a friend, while I had nothing to do my friend sat there with his computer with the screen all dark, filled with funny colourful text in lines of different length, with some lines even starting more towards the middle of the page than to the left, almost following a vertical wave pattern. He said he was writing a program to control his home remotly as well as working as a security feature that could unlock his home automatically when he got home. I was amazed by the colorful text as well as the fact that he could just create this crazy program out of nothing.
Half a year later I attended my first lecture at the computer science programme. My first program was a command line tool used for baking bread. It asked you how much flour you'd use and how many eggs, then it'd tell you wether or not you'd got the correct ratio. I was blown away by the intuitive nature of programming. I could imagine the control flow as a tree or flow chart in my head. I mean the whole program was only a couple of user inputs followed by an if-statement and a print-statement, but for me it was awe inspiring. I knew then that I'd probably chosen the right path in education. -
Am I the only developer in existence who's ever dealt with Git on Windows? What a colossal train wreck.
1. Authentication. Since there is no ssh key/git url support on Windows, you have to retype your git credentials Every Stinking Time you push. I thought Git Credential Manager was supposed to save your credentials? And this was impossible over SSH (see below). The previous developer had used an http git URL with his username and password baked in for authentication. I thought that was a horrific idea so I eventually figured out how to use a Bitbucket App password.
2. Permissions errors
In order to commit and push updates, I have to run Git for Windows as Administrator.
3. No SSH for easy git access
Here's where I confess that this is a Windows Server machine running as some form of production. Please don't slaughter me! I am not the server admin.
So, I convinced the server guy to find and install some sort of ssh service for Windows just for the off times we have to make a hot fix in production. (Don't ask, but more common than it should be.)
Sadly, this ssh access is totally useless as the git colors are all messed up, the line wrap length and window size are just weird (seems about 60 characters wide by 25 lines tall) and worse of all I can't commit/push in git via ssh because Permissions. Extremely aggravating.
4. Git on Windows hangs open and locks the index file
Finally, we manage to have Git for Windows hang quite frequently and lock the git index file, meaning that we can't do anything in git (commit, push, pull) without manually quitting these processes from task manager, then browsing to the directory and deleting the .git/index.lock file.
Putting this all together, here's the process for a pull on this production server:
Launch a VNC session to the server. Close multiple popups from different services. Ask Windows to please not "restart to install updates". Launch git for Windows. Run a git pull. If the commits to be pulled involve deleting files, the pull will fail with a permissions error. Realize you forgot to launch as Administrator. Depending on how many files were deleted in the last update, you may need to quit the application and force close the process rather than answer "n" for every "would you like to try again?" file. Relaunch Git as Administrator. Run Git pull. Finally everything works.
At this point, I'd be grateful for any tips, appreciate any sympathy, and understand any hatred. Windows Server is bad. Git on Windows is bad.10 -
Major rant incoming. Before I start ranting I’ll say that I totally respect my professor’s past. He worked on some really impressive major developments for the military and other companies a long time ago. Was made an engineering fellow at Raytheon for some GPS software he developed (or lead a team on I should say) and ended up dropping fellowship because of his health. But I’m FUCKING sick of it. So fucking fed up with my professor. This class is “Data Structures in C++” and keep in mind that I’ve been programming in C++ for almost 10 years with it being my primary and first language in OOP.
Throughout this entire class, the teacher has been making huge mistakes by saying things that aren’t right or just simply not knowing how to teach such as telling the students that “int& varOne = varTwo” was an address getting put into a variable until I corrected him about it being a reference and he proceeded to skip all reference slides or steps through sorting algorithms that are wrong or he doesn’t remember how to do it and saying, “So then it gets to this part and....it uh....does that and gets this value and so that’s how you do it *doesnt do rest of it and skips slide*”.
First presentation I did on doubly linked lists. I decided to go above and beyond and write my own code that had a menu to add, insert at position n, delete, print, etc for a doubly linked list. When I go to pull out my code he tells me that I didn’t say anything about a doubly linked list’s tail and head nodes each have a pointer pointing to null and so I was getting docked points. I told him I did actually say it and another classmate spoke up and said “Ya” and he cuts off saying, “No you didn’t”. To which I started to say I’ll show you my slides but he cut me off mid sentence and just yelled, “Nope!”. He docked me 20% and gave me a B- because of that. I had 1 slide where I had a bullet point mentioning it and 2 slides with visual models showing that the head node’s previousNode* and the tail node’s nextNode* pointed to null.
Another classmate that’s never coded in his life had screenshots of code from online (literally all his slides were a screenshot of the next part of code until it finished implementing a binary search tree) and literally read the code line by line, “class node, node pointer node, ......for int i equals zero, i is less than tree dot length er length of tree that is, um i plus plus.....”
Professor yelled at him like 4 times about reading directly from slide and not saying what the code does and he would reply with, “Yes sir” and then continue to read again because there was nothing else he could do.
Ya, he got the same grade as me.
Today I had my second and final presentation. I did it on “Separate Chaining”, a hashing collision resolution. This time I said fuck writing my own code, he didn’t give two shits last time when everyone else just screenshot online example code but me so I decided I’d focus on the PowerPoint and amp it up with animations on models I made with the shapes in PowerPoint. Get 2 slides in and he goes,
Prof: Stop! Go back one slide.
Me: Uh alright, *click*
(Slide showing the 3 collision resolutions: Open Addressing, Separate Chaining, and Re-Hashing)
Prof: Aren’t you forgetting something?
Me: ....Not that I know of sir
Prof: I see Open addressing, also called Open Hashing, but where’s Closed Hashing?
Me: I believe that’s what Seperate Chaining is sir
Prof: No
Me: I’m pretty sure it is
*Class nods and agrees*
Prof: Oh never mind, I didn’t see it right
Get another 4 slides in before:
Prof: Stop! Go back one slide
Me: .......alright *click*
(Professor loses train of thought? Doesn’t mention anything about this slide)
Prof: I er....um, I don’t understand why you decided not to mention the other, er, other types of Chaining. I thought you were going to back on that slide with all the squares (model of hash table with animations moving things around to visualize inserting a value with a collision that I spent hours on) but you didn’t.
(I haven’t finished the second half of my presentation yet you fuck! What if I had it there?)
Me: I never saw anything on any other types of Chaining professor
Prof: I’m pretty sure there’s one that I think combines Open Addressing and Separate Chaining
Me: That doesn’t make sense sir. *explanation why* I did a lot of research and I never saw any other.
Prof: There are, you should have included them.
(I check after I finish. Google comes up with no other Chaining collision resolution)
He docks me 20% and gives me a B- AGAIN! Both presentation grades have feedback saying, “MrCush, I won’t go into the issues we discussed but overall not bad”.
Thanks for being so specific on a whole 20% deduction prick! Oh wait, is it because you don’t have specifics?
Bye 3.8 GPA
Is it me or does he have something against me?7 -
My friend sent me this as WYSIWYG
/* A simple quine (self-printing program), in standard C. */ /* Note: in designing this quine, we have tried to make the code clear * and readable, not concise and obscure as many quines are, so that * the general principle can be made clear at the expense of length. * In a nutshell: use the same data structure (called "progdata" * below) to output the program code (which it represents) and its own * textual representation. */ #include <stdio.h> void quote(const char *s) /* This function takes a character string s and prints the * textual representation of s as it might appear formatted * in C code. */ { int i; printf(" \""); for (i=0; s[i]; ++i) { /* Certain characters are quoted. */ if (s[i] == '\\') printf("\\\\"); else if (s[i] == '"') printf("\\\""); else if (s[i] == '\n') printf("\\n"); /* Others are just printed as such. */ else printf("%c", s[i]); /* Also insert occasional line breaks. */ if (i % 48 == 47) printf("\"\n \""); } printf("\""); } /* What follows is a string representation of the program code, * from beginning to end (formatted as per the quote() function * above), except that the string _itself_ is coded as two * consecutive '@' characters. */ const char progdata[] = "/* A simple quine (self-printing program), in st" "andard C. */\n\n/* Note: in designing this quine, " "we have tried to make the code clear\n * and read" "able, not concise and obscure as many quines are" ", so that\n * the general principle can be made c" "lear at the expense of length.\n * In a nutshell:" " use the same data structure (called \"progdata\"\n" " * below) to output the program code (which it r" "epresents) and its own\n * textual representation" ". */\n\n#include <stdio.h>\n\nvoid quote(const char " "*s)\n /* This function takes a character stri" "ng s and prints the\n * textual representati" "on of s as it might appear formatted\n * in " "C code. */\n{\n int i;\n\n printf(\" \\\"\");\n " " for (i=0; s[i]; ++i) {\n /* Certain cha" "racters are quoted. */\n if (s[i] == '\\\\')" "\n printf(\"\\\\\\\\\");\n else if (s[" "i] == '\"')\n printf(\"\\\\\\\"\");\n e" "lse if (s[i] == '\\n')\n printf(\"\\\\n\");" "\n /* Others are just printed as such. */\n" " else\n printf(\"%c\", s[i]);\n " " /* Also insert occasional line breaks. */\n " " if (i % 48 == 47)\n printf(\"\\\"\\" "n \\\"\");\n }\n printf(\"\\\"\");\n}\n\n/* What fo" "llows is a string representation of the program " "code,\n * from beginning to end (formatted as per" " the quote() function\n * above), except that the" " string _itself_ is coded as two\n * consecutive " "'@' characters. */\nconst char progdata[] =\n@@;\n\n" "int main(void)\n /* The program itself... */\n" "{\n int i;\n\n /* Print the program code, cha" "racter by character. */\n for (i=0; progdata[i" "]; ++i) {\n if (progdata[i] == '@' && prog" "data[i+1] == '@')\n /* We encounter tw" "o '@' signs, so we must print the quoted\n " " * form of the program code. */\n {\n " " quote(progdata); /* Quote all. */\n" " i++; /* Skip second '" "@'. */\n } else\n printf(\"%c\", p" "rogdata[i]); /* Print character. */\n }\n r" "eturn 0;\n}\n"; int main(void) /* The program itself... */ { int i; /* Print the program code, character by character. */ for (i=0; progdata[i]; ++i) { if (progdata[i] == '@' && progdata[i+1] == '@') /* We encounter two '@' signs, so we must print the quoted * form of the program code. */ { quote(progdata); /* Quote all. */ i++; /* Skip second '@'. */ } else printf("%c", progdata[i]); /* Print character. */ } return 0; }6 -
Since Friday devRant posts where errors are introduced by very dumb things like commas have stood out to me.
Today's error fix. Line 1352. A string input defined in the spec file was set for 13 length. The body file had 12 dashes to represent this input.
Really, one dash, four days to solve.
Oh and Unix over Windows because my compiler on Windows didn't catch it but the Unix one sure did which is how I found it. -
If not understanding code, read the documentation or debug the code. When trying to modify...
1. Follow proper indentation.
2. Don't make spelling mistakes and follow naming convention.
3. Don't try to write all the code in one line (based on line length set)
4. Simplify if else statements if possible.
5. If value of method call need to be used once, don't store it in a variable. Directly use where ever it is needed.
6. If there is duplicated code, put it in separate method and re-use it if possible.1 -
This is gonna be a long post, and inevitably DR will mutilate my line breaks, so bear with me.
Also I cut out a bunch because the length was overlimit, so I'll post the second half later.
I'm annoyed because it appears the current stablediffusion trend has thrown the baby out with the bath water. I'll explain that in a moment.
As you all know I like to make extraordinary claims with little proof, sometimes
for shits and giggles, and sometimes because I'm just delusional apparently.
One of my legit 'claims to fame' is, on the theoretical level, I predicted
most of the developments in AI over the last 10+ years, down to key insights.
I've never had the math background for it, but I understood the ideas I
was working with at a conceptual level. Part of this flowed from powering
through literal (god I hate that word) hundreds of research papers a year, because I'm an obsessive like that. And I had to power through them, because
a lot of the technical low-level details were beyond my reach, but architecturally
I started to see a lot of patterns, and begin to grasp the general thrust
of where research and development *needed* to go.
In any case, I'm looking at stablediffusion and what occurs to me is that we've almost entirely thrown out GANs. As some or most of you may know, a GAN is
where networks compete, one to generate outputs that look real, another
to discern which is real, and by the process of competition, improve the ability
to generate a convincing fake, and to discern one. Imagine a self-sharpening knife and you get the idea.
Well, when we went to the diffusion method, upscaling noise (essentially a form of controlled pareidolia using autoencoders over seq2seq models) we threw out
GANs.
We also threw out online learning. The models only grow on the backend.
This doesn't help anyone but those corporations that have massive funding
to create and train models. They get to decide how the models 'think', what their
biases are, and what topics or subjects they cover. This is no good long run,
but thats more of an ideological argument. Thats not the real problem.
The problem is they've once again gimped the research, chosen a suboptimal
trap for the direction of development.
What interested me early on in the lottery ticket theory was the implications.
The lottery ticket theory says that, part of the reason *some* RANDOM initializations of a network train/predict better than others, is essentially
down to a small pool of subgraphs that happened, by pure luck, to chance on
initialization that just so happened to be the right 'lottery numbers' as it were, for training quickly.
The first implication of this, is that the bigger a network therefore, the greater the chance of these lucky subgraphs occurring. Whether the density grows
faster than the density of the 'unlucky' or average subgraphs, is another matter.
From this though, they realized what they could do was search out these subgraphs, and prune many of the worst or average performing neighbor graphs, without meaningful loss in model performance. Essentially they could *shrink down* things like chatGPT and BERT.
The second implication was more sublte and overlooked, and still is.
The existence of lucky subnetworks might suggest nothing additional--In which case the implication is that *any* subnet could *technically*, by transfer learning, be 'lucky' and train fast or be particularly good for some unknown task.
INSTEAD however, what has happened is we haven't really seen that. What this means is actually pretty startling. It has two possible implications, either of which will have significant outcomes on the research sooner or later:
1. there is an 'island' of network size, beyond what we've currently achieved,
where networks that are currently state of the3 art at some things, rapidly converge to state-of-the-art *generalists* in nearly *all* task, regardless of input. What this would look like at first, is a gradual drop off in gains of the current approach, characterized as a potential new "ai winter", or a "limit to the current approach", which wouldn't actually be the limit, but a saddle point in its utility across domains and its intelligence (for some measure and definition of 'intelligence').4 -
I'm sorry, but who thought eslint or any linters for that matter were a good idea.
Started a new vue project using the cli, installed tailwind... oh what's that you don't like the line length... get out of here. It's an SVG and using a class-based framework. The hell.
Any way of removing eslint? I just want to code and not get bs warnings because of an svg length or because I add one to many classes.16 -
Refactoring code to meet style guide..... 20 bucks says outside of my code reviews no one will look at it for years.
-
Did I randomly hallucinate that 74 or 75 characters used to be a popular line length limit? I've been using 74 ever since I learned what a formatter is because it was the smallest number I heard of until then, but now I can't find any source for this.
Before anyone complains, I prefer short lines whenever I get a say because my eyes are pretty bad and I want to be able to use a large font on a 14" laptop.12 -
line breaks after 100 or so characters suck. function chaining will look bad in one line, but it doesn't mean we need to limit all our lines to a hard length.
ex:
config.logger.e(TAG, "func() called with: p1 = $p1, p2 = $p2, p3 = $p3, p4 = $p4, p5 = $p5")
this is a standard log that i add at the start of my functions . its easy to autogenerate in intellij , even if my class and variable names are much long (like mVideoAnalyticsManager) . when these variables and their string names add up, they will easily go out of the screen and i won't mind scrolling a few seonds to the left to ensure everything is correct (which 99.9% is)
but a fucking ctrl+alt+l and all strings are broken with a fucking +
+
+
+
+)
AAGh its just irritating ://////4 -
As a now obsolete practice in the age of widescreen monitors and resolutions many times greater, do you still enforce line lengths in your code?
If so, what length do you use and why?7