Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
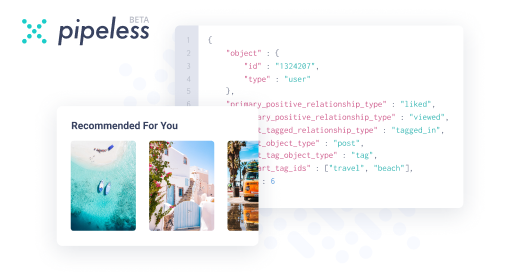
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "modulo"
-
I challenged my girlfriend (and myself) for the FizzBuzz thingy. I did it in js (because I suck at js) and she in Java. I never saw such a way to write FizzBuzz. Turns out she forgot there is a thing called modulo operator.
I know there are more elegant ways to solve it! Feel free to post your favorites.43 -
During my first-ever technical interview, the interviewer asked me "Do you know the FizzBuzz problem?"
"Uhh, not really." (I was just thinking ok this problem has a name, must be some algorithm problem)
"So the problem is basically to give you the numbers 1 to 100, if the number is divisible by 3, print 'Fizz', if divisible by 5, print 'Buzz', if divisible by 3 and 5, print 'FizzBuzz'. For other numbers just print out the number itself."
After hearing the problem, I felt so many ideas popping out of my stressed brain.
I thought for a bit and said "ok, so if the digit sum of a number is a multiple of 3, then the number is divisible by 3, and if the last digit is either 0 or 5, it's divisible by 5."
Then I started to code out my solution until the interviewer said "there's an easier solution. Can you think of it?"
This stressed me out even more.
I thought for a bit and said "well, starting from 3, keep a counter that records how many iterations are done after 3. When the counter hits 3, that number would be divisible by 3 for sure. Should I try this solution?"
The interviewer said "Sure." So I started again.
However, I struggled for about another 3min until I realized this solution is a lot harder to implement. The interviewer probably saw my struggle too.
This was the point where he stepped in and asked me "Ummmm there's an easy way of solving this. Have you heard of the MODULO OPERATOR?"
In sheer embarrassment, I finished the code in 30s.
Of course, there was no further question after this, and I felt the need to seriously reevaluate my intelligence afterwards.15 -
Chrome, Firefox, and yes even you Opera, Falkon, Midori and Luakit. We need to talk, and all readers should grab a seat and prepare for some reality checks when their favorite web browsers are in this list.
I've tried literally all of them, in search for a lightweight (read: not ridiculously bloated) web browser. None of them fit the bill.
Yes Midori, you get a couple of bonus points for being the most lightweight. Luakit however.. as much as I like vim in my terminal, I do not want it in a graphical application. Not to mention that just like all the others you just use webkit2gtk, and therefore are just as bloated as all the others. Lightweight my ass! But programmable with Lua, woo! Not like Selenium, Chrome headless, ... does that for any browser. And that's it for the unique features as far as I'm concerned. One is slow, single-threaded and lightweight-ish (Midori) and another has vim keybindings in an application that shouldn't (Luakit).
Pretty much all of them use webkit2gtk as their engine, and pretty much all of them launch a separate process for each tab. People say this is more secure, but I have serious doubts about that. You're still running all these processes as the same user, and they all have full access to the X server they run under (this is also a criticism against user separation on a single X session in general). The only thing it protects against is a website crashing the browser, where only that tab and its process would go down. Which.. you know.. should a webpage even be able to do that?
But what annoys me the most is the sheer amount of memory that all of these take. With all due respect all of you browsers, I am not quite prepared to give 8 fucking gigabytes - half the memory in this whole box! - just for a dozen or so tabs. I shouldn't have to move my web browser to another lesser used 16GB box, just to prevent this one from going into fucking swap from a dozen tabs. And before someone has a go at the add-ons, there's 4 installed and that's it. None of them are even close to this complete and utter memory clusterfuck. It's the process separation. Each process consumes half a GB of memory, and there's around a dozen of them in a usual browsing session. THAT is the real problem. And I want to get rid of it.
Browsers are at their pinnacle of fucked up in my opinion, literally to the point where I'm seriously considering elinks. Being a sysadmin, I already live my daily life in terminals anyway. As such I also do have resources. But because of that I also associate every process with its cost to run it, in terms of resources required. Web browsers are easily at the top of the list.
I want to put 8GB into perspective. You can store nearly 2 entire DVD movies in that memory. However media players used to play them (such as SMPlayer) obviously don't do that. They use 60-80MB on average to play the whole movie. They also require far less processing power than YouTube in a web browser does, even when you download that exact same video with youtube-dl (either streamed within the media player or externally). That is what an application should be.
Let's talk a bit about these "complicated" websites as well. I hate to break it to you framework web devs, but you're a dime a dozen. The competition is high between web devs for that exact reason. And websites are not complicated. The document itself is plain old HTML, yes even if your framework converts to it in the background. That's the skeleton of your document, where I would draw a parallel with documents in office suites that are more or less written in XML. CSS.. oh yes, markup. Embolden that shit, yes please! And JavaScript.. oh yes, that pile of shit that's been designed in half a day, and has a framework called fucking isEven (which does exactly what it says on the tin, modulo 2 be damned). Fancy some macros in your text editor? Yes, same shit, different pile.
Imagine your text editor being as bloated as a web browser. Imagine it being prone to crashing tabs like a web browser. Imagine it being so ridiculously slow to get anything done in your productivity suite. But it's just the usual with web browsers, isn't it? Maybe Gopher wasn't such a bad idea after all... Oh and give me another update where I have to restart the browser when I commit the heinous act of opening another tab, just because you had to update your fucking CA certs again. Yes please!19 -
Just finished an assignment yesterday where we had to code a math problem in assembler in under 20 lines
looking at the task: Hey, we already did this in Python
*building own modulo Operation for that cause we cant use it*
*freaking out when realizing it's just not posibble in 20 lines*
Then scrolling up the wikipedia page to see you've spend hours trying to implement the wrong thing3 -
!rant Eeeeeeeeee!!!! The interpreter can now handle floats and integers! And accept the power operator! And modulo! (Sorry, absurdly excited)2
-
Was reading about FizzBuzz/Algo again and I guess the modulo run-time.
The most optimal solution I came across is like:
if (x % 15) FizzBuzz
else if (x % 3) Buzz
else if (x % 5) Fizz
else x
But then how long does % take? Should you care?
I was thinking it should use variables:
var f = x % 3 == 0
var b = x % 5 == 0
if (f && b) FizzBuzz
else if (f) Fizz
else if (b) Buzz
else x10 -
During my first-ever technical interview, the interviewer asked me "Do you know the FizzBuzz problem?"
"Uhh, not really." (I was just thinking ok this problem has a name, must be some algorithm problem)
"So the problem is basically to give you the numbers 1 to 100, if the number is divisible by 3, print 'Fizz', if divisible by 5, print 'Buzz', if divisible by 3 and 5, print 'FizzBuzz'. For other numbers just print out the number itself."
After hearing the problem, I felt so many ideas popping out of my stressed brain.
I thought for a bit and said "ok, so if the digit sum of a number is a multiple of 3, then the number is divisible by 3, and if the last digit is either 0 or 5, it's divisible by 5."
Then I started to code out my solution until the interviewer said "there's an easier solution. Can you think of it?"
This stressed me out even more.
I thought for a bit and said "well, starting from 3, keep a counter that records how many iterations are done after 3. When the counter hits 3, that number would be divisible by 3 for sure. Should I try this solution?"
The interviewer said "Sure." So I started again.
However, I struggled for about another 3min until I realized this solution is a lot harder to implement. The interviewer probably saw my struggle too.
This was the point where he stepped in and asked me "Ummmm there's an easy way of solving this. Have you heard of the MODULO OPERATOR?"
In sheer embarrassment, I finished the code in 30s.
Of course, there was no further question after this, and I felt the need to seriously reevaluate my intelligence afterwards.11 -
I'm forced to learn Racket at University. What type of clusterfuck of a language is this?!
How come this shitty language doesn't even support floating point modulo and finding positions in a list...? Let alone those goddamn parentheses...7 -
So... Three of us have the task to do security reviews for one team... As to who reviews what, we decided on the basic algo of assigning an index to our names and then doing (ticket number) % 3 to get the index and therefore the reviewer for that task... Simple enough, but still you need to modulo the number and remember your index so I created a simple .html file so we can easily see who reviews which ticket by inputting the ticket number... In a hurry I named it whoreview.html
Today, the manager saw it and said to rename it before HR gets involved :D -
So I'm working on an assignment for my Computer Science class, and we have to basically compute strings into hash values and then modulo it by 1 million and put it into the hash table. But the value keeps overflowing and turning into negative values, anyone know how I can calculate the key?
(BTW, the hashcode is the same code that is calculated by the .hashCode function in Java)5 -
So for a question on Codeforces, I got the basic logic right, but for one particular test case, the input is a huge number of 250 digits. But the most unsigned long long int can handle is 19 digits. So I used double instead of int, but that makes me lose precision. And I also cannot use the % operator (modulo) which is int only. How do I get around this ?2
-
If we can transform the search space or properties of a product into a graph problem
we could possibly use Kirchhoff's theorem to reveal products which are 'low complexity'
in particular search spaces, yeah?
Now according to
https://en.wikipedia.org/wiki/...
"n Cycle Space, A family of sets closed under the symmetric difference operation can be described algebraically as a vector space over the two-element finite field Z 2 {\displaystyle \mathbb {Z} _{2}} \mathbb{Z } _{2}.[4] This field has two elements, 0 and 1, and its addition and multiplication operations can be described as the familiar addition and multiplication of integers, taken modulo 2"
Wouldn't this relate to pollards algorithm, because it involves looking for factors of coprimes modulo N or am I mistaken?
Now, according to wikipedia, "in a group, the additive identity is the identity element of the group, is often denoted 0, and is unique."
If we make the multiplicative identity of our ring or field a tuple of the ratio of a/b for some product p, or a (and a/w, where w is the square root of p), or any other set such that n*m allows us to derive a or b, we could reduce the additive identity to the multiplicative identity, making the ring trivial. Solving for p would then mean finding a function from R to R, mapping every number to 0, i.e. finding the additive identity.
Now in a system with a multiplication operation the distributes over addition, the "additive
identity annihilates ring elements", so naturally, the function that maps to 0, gives us
our additive identity, we need only find the subset, no?
Forgive me if I'm wrong, but shouldn't this be convertible to a graph search?
I'm WAY out of my depth here so if anyone is familiar and can enlighten me I'd be grateful.
It's all unknown unknowns to me.