Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
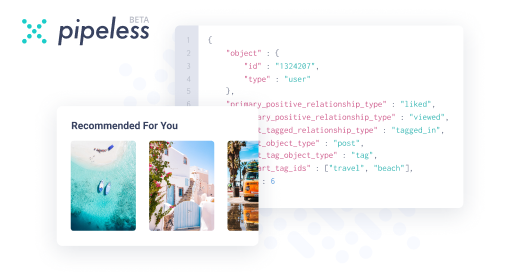
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "prng"
-
Hmm. So have you ever argued in a job interview? Like really standing your ground? In a technical interview?
Today I had a live coding session with a company I'm interested in. The developer was giving me tasks to evolve the feature on and on.
Everything was TDD. Splendid!
However at one point I had to test if the outcome of the method call is random. What I did is basically:
```
Provider<String> provider = new SomeProvider("aaa", "bbb", "ccc", "ddd", "eee", "fff")
for(int i=0; i<100; i++) {
String str = provider.get();
map.put(str, incrementCount(str));
}
Set<Integer> occurences = new HashSet(map.values());
occurences.removeIf(o -> o.equals(occurences.get(0)));
assertFalse(occurences.empty());
```
and I called it good enough, since I cannot verify true randomness.
But the dev argued that this is not enough and I must verify whether the output is truly random or not, and the output (considering the provider only has a finite set of values to return) occurences are almost equal (i.e. the deviation from median is the median itself).
I argued this is not possible and it beats the core principle of randomness -- non-determinism. Since if you can reliably test whether the sequence is truly random you must have an algorithm which determines what value can or cannot be next in the sequence. Which means determinism. And that the (P)RNG is then flawed. The best you can do is to test whether randomness is "good enough" for your use case.
We were arguing and he eventually said "alright, let's call it a good enough solution, since we're short on time".
I wonder whether this will have adverse effect my evaluation . So have you ever argued with your interviewer? Did it turn out to the better or to the worse?
But more importantly, was I right? :D21 -
I'm delirious so here's your daily dose of fuck:
```fasm
; --- * --- * ---
; 64-bit byte-by-byte mash
macro clamp_u8 {
mov cl,$08;
mov rdx,rax;
rept 8 \{
rol rdx,cl;
xor al,dl;
\};
};
; --- * --- * ---
; give 8-bit random seed
macro prng_u8 {
rdtsc;
shl rdx,32;
or rax,rdx;
clamp_u8;
};
; --- * --- * ---
; roll dice
d20: prng_u8;
; x%20, according to gcc ;>
mov edi,eax;
mov eax,-51;
mul dil;
shr ax,12;
lea eax,[rax+rax*4];
lea edx,[0+rax*4];
mov eax,edi;
sub eax,edx;
; discard high and give
and rax,$FF;
ret;
```
I guess `d20` could be inlined too but I thought it'd be too much.
Is it faster than straight C? Probably not. But it's way lighter, so it loads faster. Below five hundred bytes mother fucker.
Now if you'll excuse me, I'll go sit in the darkness repeteadly typing roll 1d20 on the terminal. For reasons.9 -
I am looking for new programming language to learn and I found D language. It looks interesting but I think that community is weak and there is lack of some usefull libraries.
For example I can not find secure PRNG.
So my questions are:
> Have you any experience with this language?
> It is worth to learn?3 -
!rant
This is neat https://twitter.com/atlasobscura/... take a picture of bunch of lava lamps, make that bit array your entropy for crypto.1 -
I know it's a bit late from the official launch of iOS 11, but finally I get to have my hands on this. So, let's jump in and explore what's new.11
-
An article on generating random sequences with few gaps:
https://quantamagazine.org/oxford-m...
I wonder what the entropy of such sequences are, and if these sequences would be suitable for the basis of generating secure random numbers, or at least useful as a PRNG? -
Let's say I take a matrix of high entropy random numbers (call it matrix J), and encode a problem into those numbers (represented as some integers which in turn represent some operations and data).
And then I generate *another* high entropy random number matrix (call it matrix K). As I do this I measure the Pearson's correlation coefficient between J (before encoding the problem into it, call Jb), and K, and the correlation between J (after encoding, let's call it Ja) and K.
I stop at some predetermined satisfactory correlation level, let's say > 0.5 or < -0.5
I do this till Ja is highly correlated with some sample of K, and Jb's correlation with K is close to 0.
Would the random numbers in K then represent, in some way, the data/problem encoded in Ja? Or is it merely a correlation?
Keep in mind K has no direct connection to J, Ja, or Jb, we're only looking for a matrix of high entropy random numbers that indicated a correlation to J and its data.
I say "high entropy", it would be trivial to generate random numbers with a PRNG that are highly correlated simply by virtue of the algorithm that generated them.12