Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
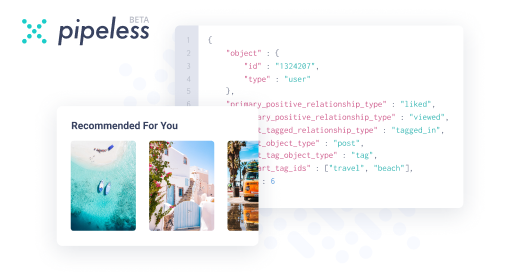
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "single js file"
-
!rant
This was over a year ago now, but my first PR at my current job was +6,249/-1,545,334 loc. Here is how that happened... When I joined the company and saw the code I was supposed to work on I kind of freaked out. The project was set up in the most ass-backward way with some sort of bootstrap boilerplate sample app thing with its own build process inside a subfolder of the main angular project. The angular app used all the CSS, fonts, icons, etc. from the boilerplate app and referenced the assets directly. If you needed to make changes to the CSS, fonts, icons, etc you would need to cd into the boilerplate app directory, make the changes, run a Gulp build that compiled things there, then cd back to the main directory and run Grunt build (thats right, both grunt and gulp) that then built the angular app and referenced the compiled assets inside the boilerplate directory. One simple CSS change would take 2 minutes to test at minimum.
I told them I needed at least a week to overhaul the app before I felt like I could do any real work. Here were the horrors I found along the way.
- All compiled (unminified) assets (both CSS and JS) were committed to git, including vendor code such as jQuery and Bootstrap.
- All bower components were committed to git (ALL their source code, documentation, etc, not just the one dist/minified JS file we referenced).
- The Grunt build was set up by someone who had no idea what they were doing. Every SINGLE file or dependency that needed to be copied to the build folder was listed one by one in a HUGE config.json file instead of using pattern matching like `assets/images/*`.
- All the example code from the boilerplate and multiple jQuery spaghetti sample apps from the boilerplate were committed to git, as well as ALL the documentation too. There was literally a `git clone` of the boilerplate repo inside a folder in the app.
- There were two separate copies of Bootstrap 3 being compiled from source. One inside the boilerplate folder and one at the angular app level. They were both included on the page, so literally every single CSS rule was overridden by the second copy of bootstrap. Oh, and because bootstrap source was included and commited and built from source, the actual bootstrap source files had been edited by developers to change styles (instead of overriding them) so there was no replacing it with an OOTB minified version.
- It is an angular app but there were multiple jQuery libraries included and relied upon and used for actual in-app functionality behavior. And, beyond that, even though angular includes many native ways to do XHR requests (using $resource or $http), there were numerous places in the app where there were `XMLHttpRequest`s intermixed with angular code.
- There was no live reloading for local development, meaning if I wanted to make one CSS change I had to stop my server, run a build, start again (about 2 minutes total). They seemed to think this was fine.
- All this monstrosity was handled by a single massive Gruntfile that was over 2000loc. When all my hacking and slashing was done, I reduced this to ~140loc.
- There were developer's (I use that term loosely) *PERSONAL AWS ACCESS KEYS* hardcoded into the source code (remember, this is a web end app, so this was in every user's browser) in order to do file uploads. Of course when I checked in AWS, those keys had full admin access to absolutely everything in AWS.
- The entire unminified AWS Javascript SDK was included on the page and not used or referenced (~1.5mb)
- There was no error handling or reporting. An API error would just result in nothing happening on the front end, so the user would usually just click and click again, re-triggering the same error. There was also no error reporting software installed (NewRelic, Rollbar, etc) so we had no idea when our users encountered errors on the front end. The previous developers would literally guide users who were experiencing issues through opening their console in dev tools and have them screenshot the error and send it to them.
- I could go on and on...
This is why you hire a real front-end engineer to build your web app instead of the cheapest contractors you can find from Ukraine.19 -
TABLE BASED WEB DESIGN
I was surprised there were no rants about this topic before I realized it was more than a decade back 😳
We've never had it better! So to help add a little perspective for all those ranting about what is unarguably the golden age for web developers... let me fill you in on web dev in the late 90's;
JavaScript was a joke. No seriously! - I once got laughed out of the room for suggesting we try use it for more than disabling a button - (I wanted to check out the new XHR request thingy [read AJAX]).
HTML was simple and purely a markup language (with the exception of the marquee tag). The tags were basically just p,ul,ol,h*,form inputs,img and table and html took 10 minutes to learn. Any style was inline and equally crude - anything that wasn't crude could not be trusted and probably wouldn't render at all in most browsers (never mind render correctly).
There were rumors of a style TAG and something called a cascading style sheet which were received with much skepticism since it went against the old ways and any time saved would be lost writing multiple [IE version specific] style sheets for each browser just to get it to work - so we simply didn't.
No CSS meant the only tags you had to work with to create a structured layout were br, hr and table... so naturally EVERYTHING was in nested tables! JS callback hell can't touch this! - it was not uncommon to have 50+ nested tables all with inline style in a single page which would be edited without any dev tools or linting.
You would spend 30 minutes scanning td tags until your eyes bled to find something, make a change, ftp the file to the server, reload the web page and then spend 10 minutes staring at the devastation on your screen convinced you broke
the internet before spotting an un-closed td tag with your bloodshot eyes.
Tables were not just a silver bullet - they were the ONLY bullet and were in the wild west!
Q: Want an inline form or to align your inputs left?
A: Duh table!
Q: Want a border with round-corners, a shadow or blur?
A: That's easy! Your gonna want to put that table in the center cell of another table then crop a image of the border into 6 smaller images to put in the surrounding cells... oh and then spend 10 minutes fucking with mystical attributes like cell-padding and valign to get them flush.
...But hey at least on the bright-side vertically & horizontally centering stuff was a breeze!22 -
Honestly, I’ve been complaining a lot about the company I joined a few months ago and especially about the code base and I just have to say ...
With good fucking reason, look at this shit, every single *.js file of our Angular app is manually added inside the master page
Not even minimized what the actual fuckkkkkkkkkkk15 -
Somebody asked on how to get started on Full Stack web application development.
This is how I got started.
Client side Web Application Development:
---------------------------------------------------------------
• Start with basic HTML, CSS and JS, JSON. For quick learning, see W3Schools for these topic or YouTube it.
• Get a local web server. "200 OK!" webserver chrome extension is a good start. (https://chrome.google.com/webstore/...)
• Learn Chrome Dev Tools to debug the pages. YouTube it.
• Get a good IDE. I am very happy with VSCode. You can use it for very serious WebApps.
• Start learning JavaScript language in depth, but just related to Web Browser related topic or you would get sucked in server side too early.
• Install node.js. Learn NPM package manager. Learn basic node commands.
• Learn complexity of JS file referencing, JS modules in browser. Just learn, don't use it yet, to understand the benefits of code bundlers.
• Learn Webpack code bundler.
• Learn how to make you simple site much faster and using in Mobile using "Progressive Web Apps".
• Now learn to make modular UIs. I love React. Focus on getting the UI code modulear. Create Single Page sites. (You are not there yet to create a Web App) “Create-React-App” started kit is a good starting point.
• Learn to create multi-page site using React-router.
• Learn application state management using Redux.
• Learn to create application decision engine using Redux-Saga.
Practice and master each stage.
Along above, learn git / GitHub (to learn from others code), find good web resources like Medium / Smashing magazine, good YouTube channels etc. I subscribed to some popular Udemy courses too.
Server side Web development:
------------------------------------------
:) First learn client side Web Application development. Server side learning is another story.3 -
So, after weeks of reading spicy rants from all of you, I finally decided to join your community ; even if I'm only a student, I've encountered some solid crap in my internships.
Let's go back in time bois. Two years ago, I started my first intership at a Fortune 500 company (this doesn't exists in France, but whatever, this is nearly the same category). I was supposed to build some file sharing system for the office. Before getting into it, I briefly thought aboyt what technos I could use to build it and make a sweet interface for my co-workers, in 10 weeks, and not a single another day.
Expectations
> Nice team with devs that I could ask things about and learn solid tricks that would even amaze David Copperfield
> Having a nice dev environment
Reality
> Alone on this project
> No fucking dev environment, I had to build everything on Notepad
> No CI
> No SCM
> And, the worst, Ladies and Gentlemans,
I FUCKING HAD TO WORK IN A SINGLE FILE IN A CLOSED ENVIRONMENT.
NO WEBSERVER, NO DEDICATED SPACE.
I HAD TO REQUEST A SPECIFIC ENVIRONMENT IN A CLOSED CUSTOM CMS THAT WAS SERVING FILES, SO THIS FORMAT COULD BE READ ON FOLDER OPENING IN IE9 (FIREFOX FORBIDDEN).
YOU HAD TO MIX HTML, CSS AND JS IN A SINGLE FILE. NO SERVER-SIDE LANGUAGES, ONLY STATIC LINKS, NO FRAMEWORKS (if we can call jQuery, Bootstrap, Semantic UI and all these thinks "Frameworks").
> mfw at the end of the intership13 -
I think I want to quit my first applicantion developer job 6 months in because of just how bad the code and deployment and.. Just everything, is.
I'm a C#/.net developer. Currently I'm working on some asp.net and sql stuff for this company.
We have no code standards. Our project manager is somewhere between useless and determinental. Our clients are unreasonable (its the government, so im a bit stifled on what I can say.) and expect absurd things from us. We have 0 automated tests and before I arrived all our infrastructure wasn't correct to our documentation... And we barely had any documentation to begin with.
The code is another horror story. It's out sourced C# asp.net, js and SQL code.. And to very bad programmers in India, no offense to the good ones, I know you exist. Its all spagheti. And half of it isn't spelled correctly.
We have a single, massive constant class that probably has over 2000 constants, I don't care to count. Our SQL projects are a mess with tons of quick fix scripts to run pre and post publishing. Our folder structure makes no sense (We have root/js and root/js1 to make you cringe.) our javascript is majoritly on the asp.net pages themselves inline, so we don't even have minification most of the time.
It's... God awful. The result of a billion and one quick fixes that nobody documented. The configuration alone has to have the same value put multiple times. And now our senior developer is getting the outsourced department to work on moving every SINGLE NORMAL STRING INTO THE DATABASE. That's right. Rather then putting them into some local resource file or anything sane, our website will now be drawing every single standard string from the database. Our SENIOR DEVELOPER thinks this is a good idea. I don't need to go into detail about how slow this is. Want to do it on boot? Fine. But they do it every time the page loads. It's absurd.
Our sql database design is an absolute atrocity. You have to join several tables together just to get anything done. Half of our SP's are failing all the time because nobody really understands the design. Its gloriously awful its like.. The epitome of failed database designs.
But rather then taking a step back and dealing with all the issues, we keep adding new features and other ones get left in the dust. Hell, we don't even have complete browser support yet. There were things on the website that were still running SILVERLIGHT. In 2019. I don't even know how to feel about it.
I brought up our insane technical debt to our PM who told me that we don't have time to worry about things like technical debt. They also wouldn't spend the time to teach me anything, saying they would rather outsource everything then take the time to teach me. So i did. I learned a huge chunk of it myself.
But calling this a developer job was a sick, twisted joke. All our lives revolve around bugnet. Our work is our BN's. So every issue the client emails about becomes BN's. I haven't developed anything. All I've done is clean up others mess.
Except for the one time they did have me develop something. And I did it right and took my time. And then they told me it took too long, forced me to release before it was ready, even though I had never worked on what I was doing before. And it worked. I did it.
They then told me it likely wouldn't even be used anyway. I wasn't very happy at all.
I then discovered quickly the horrors of wanting to make changes on production. In order to make changes to it, we have to... Get this
Write a huge document explaining why. Not to our management. To the customer. The customer wants us to 'request' to fix our application.
I feel like I am literally against a wall. A huge massive wall. I can't get constent from my PM to fix the shitty code they have as a result of outsourcing. I can't make changes without the customer asking why I would work on something that doesn't add something new for them. And I can't ask for any sort of help, and half of the people I have to ask help from don't even speak english very well so it makes it double hard to understand anything.
But what can I do? If I leave my job it leaves a lasting stain on my record that I am unsure if I can shake off.
... Well, thats my tl;dr rant. Im a junior, so maybe idk what the hell im talking about.rant code application bad project management annoying as hell bad code c++ bad client bad design application development16 -
I’m back for a fucking rant.
My previous post I was happy, I’ve had an interview today and I felt the interviewer acted with integrity and made the role seem worthwhile. Fuck it, here’s the link:
https://www.devrant.io/rants/889363
So, since then; the recruiter got in touch: “smashed it son, sending the tech demo your way, if you can get it done this evening that would be amazing”
Obviously I said based on the exact brief I think that’s possible, I’ll take a look and let them know if it isn’t.
Having done loads of these, I know I can usually knock them out and impress in an evening with no trouble.
Here’s where shit gets fucked up; i opened the brief.
I was met with a brief for an MVP using best practice patterns and flexing every muscle with the tech available...
Then I see the requirements, these fucking dicks are after 10 functional requirements averaging an hour a piece.
+TDD so * 1.25,
+DI and dependency inversion principle * 1.1
+CI setup (1h on this platform)
+One ill requirement to use a stored proc in SQL server to return a view (1h)
+UX/UI design consideration using an old tech (1-2h)
+unobtrusive jquery form post validation (2h)
+AES-256 encryption in the db... add 2h for proper testing.
These cunts want me to knock 15-20h of Work into their interview tech demo.
I’ve done a lot of these recently, all of them topped out at 3h max.
The job is middling: average package, old tech, not the most exciting or decent work.
The interviewer alluded to his lead being a bit of a dick; one of those “the code comes first” devs.
Here’s where shit gets realer:
They’ve included mock ups in the tech demo brief’s zip... I looked at them to confirm I wasn’t over estimating the job... I wasn’t.
Then I looked at the other files in the fucking zip.
I found 3 of the images they wanted to use were copyright withheld... there’s no way these guys have the right to distribute these.
Then I look in the font folder, it’s a single ttf, downloaded from fucking DA Font... it was published less than 2mo ago, the license file had been removed: free for Personal, anything else; contact me.
There’s no way these guys have any rights to this font, and I’ve never seen a font redistributed legally without it’s accompanying licence files.
This fucking company is constantly talking about its ethical behaviours.
Given that I know what I’m doing; I know it would have taken less time to find free-for-commercial images and use a google font... this sloppy bullshit is beyond me.
Anyway, I said I’d get back to the recruiter, he wasn’t to know and he’s a good guy. I let him know I’d complete the tech demo over the weekend, he’s looked after me and I don’t want him having trouble with his client...
I’ll substitute the copyright fuckery with images I have a license for because there’s no way I’m pushing copyright stolen material to a public github repo.
I’ll also be substituting the topic and leaving a few js bombs in there to ensure they don’t just steal my shit.
Here’s my hypotheses, anyone with any more would be greatly welcomed...
1: the lead dev is just a stuck up arsehole, with no real care for his work and a relaxed view on stealing other people’s.
2: they are looking for 15-20h free work on an MVP they can modify and take to market
3: they are looking for people to turn down this job so they can support someone’s fucking visa.
In any case, it’s a shit show and I’ll just be seeing this as box checking and interview practice...
Arguments for 1: the head told me about his lead’s problems within 20mn of the interview.
2: he said his biggest problem was getting products out quickly enough.
3: the recruiter told me they’d been “picky”, and they’re making themselves people who can’t be worked for.
I’m going to knock out the demo, keep it private and protect my work well. It’s going to smash their tits off because I’m a fucking great developer... I’ll make sure I get the offer to keep the recruiter looked after.
Then fuck those guys, I’m fucking livid.
After a wonderful interview experience and a nice introduction to the company I’ve been completely put off...
So here’s the update: if you’re interviewing for a shitty middle level dev position, amongst difficult people, on an out of date stack... you need people to want you, don’t fuck them off.
If they want my time to rush out MVPs, they can pay my day rate.
Fuuuuuuuuck... I typed this out whilst listening to the podcast, I’m glad I’m not the only one dealing with shit.
Oh also; I had a lovely discriminatory as fuck application, personality test and disability request email sent to me from a company that seems like it’s still in the 90s. Fuck those guys too, I reported them to the relevant authorities and hope they’re made to look at how morally reprehensible their recruitment process is. The law is you don’t ask if the job can be done by anyone.6 -
!rant
I used to doubt the usefulness of regex, until now.
I'm new to web dev, and downloaded a sample website to make a project with, but all the sources of images came as src="images/image.jpg", and for some reason I couldn't make it work, the only way that I found that could work to me was creating a static folder inside my app folder, declaring in the start of the document a {% load static %} and referring the image source as {% static 'images/image.jpg' %} in the html file, I kinda get what this is doing, but why it's the only way that works, it's beyond me.
Great! Now I can start the development server and see the website in its full glory!!! Then I realized: I had to edit the sources of every image and every reference to css and js in 5 html files to it work properly, and come on, do all that by hand?
Then regex came to mind, never had used it, never knew how to use it properly, after some web research I found if I did a find/replace with ([a-z]\w+\/[a-z,-]+\.[a-z]+{1,2}) and {% static '$1' %}, all the work I had to do, was resumed to a single click of replace all.
Man, I love doing what I do, and I love you guys/gals, never tough I would ever find a place in which I could share this kind of thing!6 -
Fire your whole fucking web team Bethesda
* Your design is a classic ipecac. Whatever the fuck you are doing doesn't in frontend doesn't justify the 4Mb of bandwidth I wasted on a single js file. Why the fuck can I see the whole fucking node_modules directory when looking at the sources?
I know this is supposed to be a webpage for a game development studio, but I'm seriously wondering if your budget would even get me a prostitute.
I'm a greedy fuck and want a free game. apparently your servers are only good enough to register me, but login is apparently too much to ask for. Yeah sure. Oh and also thank you for choosing an "incorrect username and password" error message by default, even though your fucking gateway timed out. Please be kind enough and punch me directly into my face next time. Not like I'll ever access that shit ever again3 -
Just created a custom html, css, js bundler to bundle all my files and minify it to a single file.
Development will be a breeze right now.2 -
A long long time ago ( 2007 I think ) I worked for a company that made landing sites, so basically an email campaign would go out, users would be sent to a 1 page website with a form to capture their data, ready to be spammed even more. You know how it was back then.
So I worked with a guy who we had just hired, I didn't do the hiring but his CV checked out, so I gave him one of my tasks. Now most pages were made with js and html, with a PHP backend ( called with Ajax). Now this guy didn't know PHP so I was like all good, ASP works too at the end of the day we don't judge, we do like 2 or 3 of these a day and never look at them again. So he goes of and does is thing.
3 weeks later, the customer calls up to me they still haven't received their landing page. Ok so he probably forgot to email the customer np, I tell him to double check he has emailed the customer. Another week goes by end the customer calls back, same problem. At this point I'm getting worried, because we're days away from the deadline and it was originally my task.
So I go back to the guy and I tell him I want that landing page so I can send it myself, half thinking to myself that we had a freeloader, that guy that comes in to companies for 3 weeks, doesn't work, but still cashes his pay. But no, this was much worse.
So he tells me he has finished yet. I ask him why, what's the blocker ? You had 4 weeks to tell me you were blocked and couldn't progress. And his answer was simply, because I wasn't blocked I have been working on it this whole time. So I tell him to zip his project up and email it to me. We didn't do SVN or git back then, simply wasn't worth it. So he comes back to me and says the email server is telling him attachments can't be bigger then 50mb. At this point I'm thinking he didn't properly sized the art or something, so I give him a flash drive to put it on.
When I then open the flash drive, the archive is 300mb, thinking to myself, the images weren't even that big to begin with.
So I open it up, and I don't even find any images, just a single asp page. About 500mb. When I opened that up and it finally loaded, I saw the most horrendous things ever.
The first 500 lines was just initializing empty vars. Then there was some code that created an empty form with an onChange event that submits the form. After that.. it was just non stop nested if's. No loops, no while, for, foreach, NO elseif's, just nested if's, for every possible combination of the state the form could be in. Abou 5000 of them, in a single file. To make matters worse, all the form ( and page ) layout was hardcoded in the if's. Includes inline css, base64 encoded images, nothing but as dynamic, based on the length of the form he changes the layout, added more background etc. He cut the images up for every possible size of the page and included them in the code.
I showed it to my boss, he fired the guy on the spot. I redid the work from scratch, in under 4 hours. Send it to the client. they had no ammends to make, happy as Larry. Whish I kept the code somewhere.
Morale of the story, allways do a coding test on interviews, even if small things just to sanity check.3 -
Okay, it's FUCKing rant time.
FUCK single-file *cough* page.tpl.php *cough* drupal-sites
I FUCKing hate sites without any FUCKing structure, where all logic is built into the overall wrapping pageview file.
Spend more FUCKing time than healthy finding this golden nugget.
In a FUCKing 2000+ lines long file, in a FUCKing mix of inline CSS/JS, PHP/SQL and FUCKing exec(); calls.
Definetily the best FUCKing way to destroy a FUCKing lightbox, for people who are not logged in...
- Why would you even do that in the first FUCKing place ?!??! The customer didn't ask for this..
All this FUCKing mess because the previous developer decided to quit, and did not FUCKing care for the next maintainer to come.
Fellow drupal developers will know the struggle.3 -
Can we please stop making everything in javascript? Pretty please?
Like, I get it, js makes it easier to find developers and speeds up development, but writing a compiler to run on node? That doesn't make any sense to me. Typescript is taking ~11s to compile a medium-sized project, out of which ~9s are just type-checking (if I disable type-checking it takes ~2s to compile). Even Rust, which is considered to have a slow compiler (because of the borrow checker I assume) takes less time to compile a project of roughly the same size.
This is getting very irritating. If I want to keep type-checking, every single time I make a change on a single file I have to wait 11s before any tests start running. This was heavily affecting my productivity (and sanity), so I decided to disable type-checking and threw out the window 90% of Typescript's advantages.
Why the hell wasn't typescript written in an actually performant language? Just so you could say that typescript is written in itself? I don't get it, I really don't.13 -
Sharing a first look at a prototype Web Components library I am working on for "fun"
TL;DR left side is pivot (grouped) table, right side is declarative code for it (Everything except the custom formatting is done declaratively, but has the option to be imperative as well).
====
TL;DR (Too long, did read):
I'm challenging myself to be creative with the cool new things that browsers offer us. Lani so far has a focus on extreme extensibility, abstraction from dependencies, and optional declarative style.
It's also going to be a micro CSS framework, but that's taking the back-seat.
I wanted to highlight my design here with this table, and the code that is written to produce this result.
First, you can see that the <lani-table> element is reading template, data, and layout information from its child elements. Besides the custom highlighting code (Yellow background in the "Tags" column, and green gradient in the "Score" column), everything can be done without opening even a single script tag.
The <lani-data-source> element is rather special. It's an abstraction of any data source, and you, as a developer can add custom data sources and hook up the handlers to your whim (the element itself uses the "type" attribute to choose a handler. In this case, the handler is "download" which simply sends a fetch request to the server once and downloads the result to memory).
Templates are stored in an html file, not string literals (Which I think really fucks the code) and loaded async, then cached into an object (so that the network tab doesn't get crowded, even if we can count on the HTTP cache). This also has the benefit of allowing me to parse the HTML templates once and then caching the parsed result in memory, so templates are never re-parsed from string no matter how many custom elements are created.
Everything is "compiled" into a single, minified .js file that you include on your page.
I know it's nothing extraordinary, but for something that doesn't need to be compiled, transpiled, packaged, shipped, and kissed goodnight, I think it's a really nice design and I hope to continue work on it and improve it over time1 -
I just started using a new React component library
https://ant.design
apparently they decided that rather than include all the icons as a separate font file, or dynamically loaded SVG, they would encode every single icon as a SVG in a JS string, and concatenate them all together into a single file.
I feel dirty but I just committed a 2MB javascript payload to the staging server.
Suggestions for a LIGHTWEIGHT React/Typescript component library?1 -
To the guys that develop any form of hybrid applications, is there literally no js templating engine that accepts template files as its base? I could write probably a single file that just returns the template and then all the others request it before templating, but seriously, is there no ready to go solution?5
-
personal projects, of course, but let's count the only one that could actually be considered finished and released.
which was a local social network site. i was making and running it for about three years as a replacement for a site that its original admin took down without warning because he got fed up with the community. i loved the community and missed it, so that was my motivation to learn web stack (html, css, php, mysql, js).
first version was done and up in a week, single flat php file, no oop, just ifs. was about 5k lines long and was missing 90% of features, but i got it out and by word of mouth/mail is started gathering the community back.
right as i put it up, i learned about include directive, so i started re-coding it from scratch, and "this time properly", separated into one file per page.
that took about a month, got to about 10k lines of code, with about 30% of planned functionality.
i put it up, and then i learned that php can do objects, so i started another rewrite from scratch. two or three months later, about 15k lines of code, and 60% of the intended functionality.
i put it up, and learned about ajax (which was a pretty new thing since this was 2006), so i started another rewrite, this time not completely from scratch i think.
three months later, final length about 30k lines of code, and 120% of originally intended functionality (since i got some new features ideas along the way).
put it up, was very happy with it, and since i gathered quite a lot of user-generated data already through all of that time, i started seeing patterns, and started to think about some crazy stuff like auto-tagging posts based on their content (tags like positive, negative, angry, sad, family issues, health issues, etc), rewarding users based on auto-detection whether their comments stirred more (and good) discussion, or stifled it, tracking user's mental health and life situation (scale of great to horrible, something like that) based on the analysis of the texts of their posts...
... never got around to that though, missed two months hosting payments and in that time the admin of the original site put it back up, so i just told people to move back there.
awesome experience, though. worth every second.
to this day probably the project i'm most proud of (which is sad, i suppose) - the final version had its own builtin forum section with proper topics, reply threads, wysiwyg post editor, personal diaries where people could set per-post visibility (everyone, only logged in users, only my friends), mental health questionnaires that tracked user's results in time and showed them in a cool flash charts, questionnaire editor where users could make their own tests/quizzes, article section, like/dislike voting on everything, page-global ajax chat of all users that would stay open in bottom right corner, hangouts-style, private messages, even a "pointer" system where sending special commands to the chat aimed at a specific user would cause page elements to highlight on their client, meaning if someone asked "how do i do this thing on the page?", i could send that command and the button to the subpage would get highlighted, after they clicked it and the subpage loaded, the next step in the process would get highlighted, with a custom explanation text, etc...
dammit, now i got seriously nostalgic. it was an awesome piece of work, if i may say so. and i wasn't the only one thinking that, since showing the page off landed me my first two or three programming jobs, right out of highschool. 10 minutes of smalltalk, then they asked about my knowledge, i whipped up that site and gave a short walkthrough talking a bit about how the most interesting pieces were implemented, done, hired XD
those were good times, when I still felt like the programmer whiz kid =D
as i said, worth every second, every drop of sweat, every torn hair, several times over, even though "actual net financial profit" was around minus two hundred euro paid for those two or three years of hosting. -
!rant, more of an incredulous/cruelly amused "you had ONE job..."
so: biggest IT/PC/electronics store in my (and neighboring) country. their webpage, of course with the function to buy online, because of course.
the big green "Buy" button does nothing. doesn't work. doesn't react. I keep clicking it multiple times, shorter, longer, etc, because maybe their JS scripts are just shit so they slow.
nope.
okay. open devtools, JS console.
hover over the button: "Error: isMobile is not a function".
click the button: "Error: isMobile is not a function"
WAT.
search for isMobile in the script.
173 occurences.
fuck this.
console: isMobile = function(){return false;}
because I'm not on my phone.
click the "Buy" button.
works flawlessly.
...HOW?
THE WHOLE PAGE IS AN ESHOP YOU COMIC RELIEF INCOMPETENTS! =D
173 uses of non-existing function that blocks business-critical feature, THE ONLY CORE FEATURE FOR WHICH YOUR SITE EVEN EXISTS, and NOBODY, not the dev who fucked it up, NOT EVEN QA, noticed it??? =D =D
if I was the boss of the devs, or even boss of the whole company...
git blame
...and then i'd go the whole chain from the dev who caused the bug, through all of the QA people who "tested" that version before deploy, and I would personally, on the spot, fire each and every single one of them.
mainly because of who knows how much money this stupid not even a proper bug lost them.
but secondarily, because clearly none of those people give a single shit (n)or have an idea how to do their jobs.
=D =D
yeah but I was a good guy, filed a bug report in the "Complaints" section of their Contact form.
it goes to some call-center-like peon, so it starts with a sentence "forward this to your site's dev people outright to file as a bug, thank you".
but... HOW.... =D
HOW can you let something like this through? =D
the bottleneck of your whole user interaction, which forms first of the three steps OF THE MAIN AND MOST IMPORTANT FUNCTION of your whole business... =D
...I...
...does not compute =D
...BUT THEY USING ANGULAR, SO THEY ALL MODERN AND HIGH-TECH AND EVERYTHING'S FINE!!! =D =D1 -
What are your opinions of single file components in Vue? Will it actually help maintainability down the road for our codebase? Or do you think it’s better to separate the .js, .sass, and .vue?9
-
Pretty niche tool, but Sencha Architect!
It is a wanna be GUI-Builder/IDE for ExtJS, but neither works properly.
This rant is not about ExtJS, just about Sencha Architect, which my coworkers and I were forced to use.
If you want to join the ride, here an excerpt of just some of the issues:
- installation: already the setup is more of a gamble than an actual setup, either it works on your machine or it doesn't, plain and simple
- GUI Builder: just drag and dropping components is actually nice, but the editing capabilities are frustrating, you can't edit the UI code by hand at all, just through pre defined properties. If there was the need to really mix things up it wasn't possible, I couldn't even rebuild shown examples of their ExtJS documentation. Furthermore the property editor was data type locked, which means if you want to enter a string which ExtJS already supports, but architect locks the value as a boolean, you can't edit it at all, while still using Architect
- code editing: well it is a colored texteditor, which is fine, and I could live with that, but Architect let's you just edit areas where it allows you to - want to change something else? Nope not allowed
- autocompletion: there is none at all, same goes for refactoring, multi highlighting, string replacement, and others
- code storing: well now some may think edit it somewhere else, well no, also not possible... Architect not just only saves simple js, there is also a Json formatted file for everything you have created, which is needed so the tool can actually load it for further editing. They possibly never heard of DRY. But the worst of this code storing was actually using git along with it - have a merge conflict? Merge both files! Every single time, it was so damn tedious
There are a few more, but these were the worst I can remember.
Luckily I don't have to use it anymore!
Maybe they have fixed or changed a lot of it, because the developers were aware of the issues and eager to resolve them, as far as I was told on a roadmap presentation. And some of the tools they had released in the end of my time using ExtJS were actually really good, like an IDE plugin for the framework, and I liked using it. -
Gf asked me to help her with getting science articles. She had some page that her university suggested students to use, but had troubles with downloading documents.
At first I was like "Hey, it says use IE, other browsers are not supported. Thats bad but.. whatever". Then it popped that she needs Java enabled - well, I guess we have to... Even updated it cause it was needed.
Restarted IE, clicked download again and... Java security blocked web app... Eh, I don't trust it but whatever, just let's check what if I whitelist it.
Got some basic view, 1 dropdown list for "file name format" (like anybody cares), path selection where to save file, and some checkbox. Lame, but let's just leave it behind.
Downloaded, it turned out to be html file, not pdf, fishy that it was single file, but hoped for some text styled with css, so I opened it and got redirected to page where I clicked download.
Checked that file content - html with empty body and script tag containing js that redirects on load.
Srsly?😐2 -
Really need to make it a habit to read every single piece of documentation and included read me file for a plugin and framework that I'm using even if they essentially say the exact same thing...wasted so much time just to find out I literally needed 1 line of js instead of all kinds of custom code -_-